Setup Guide
Setting up the Flyy SDK in your Flutter project
Initialize SDK
The following guide provides steps to initialize the Flyy SDK for your Flutter Project. Please follow all the steps carefully.
Note
Complete the Firebase setup for the project before integrating the Flyy SDK.
Add Dependencies
Step 1: Depend on it
Add the following line of code in your pubspec.yaml file under dependencies.
dependencies:
...
#Without the caret ^
flyy_flutter_plugin: 1.1.105
Step 2: Install it
$ flutter pub get
Android Native Setup
Step 1: Add Auth Token
To access Flyy's private JitPack library, add this line in your project's gradle.properties file (your_project/android/gradle.properties):
authToken=jp_toc9ldlr81jv1ncq2gp2e6q1sg
Step 2: Add JitPack Repository
In your project level build.gradle file (your_project/android/build.gradle), add the maven repository inside the allprojects
closure:
buildscript {
...
}
allprojects {
repositories {
...
// Add the bellow lines
maven {
url 'https://jitpack.io'
credentials { username authToken }
}
}
}
Gradle 7.0+
If the
allprojects
closure does not exist, in your project's settings.gradle file (your_project/settings.gradle), add the maven repository:dependencyResolutionManagement { repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) repositories { ... maven { url 'https://jitpack.io' credentials { username authToken } } } }
Step 3: Edit module-level build.gradle
Edit the following parameters in the module-level build.gradle (your_project/android/app/build.gradle) within the defaultConfig
closure.
defaultConfig {
...
minSdkVersion 19
targetSdkVersion 30
...
multiDexEnabled true
}
iOS Native Setup
Step 1: Edit Podfile
Navigate to the ios folder of your project (your_project/ios) and open the Podfile file. Change the Global platform for your project to at least 11.0.
# Uncomment this line to define a global platform for your project
platform :ios, '11.0'
Step 2: Edit AppDelegate.swift
After successful pod installation, open your ios xcworkspace project in Xcode. In AppDelegate.swift inside the application(_:didFinishLaunchingWithOptions:)
method add the following lines of code.
import UIKit
import Flutter
@UIApplicationMain
@objc class AppDelegate: FlutterAppDelegate {
var navigationController = UINavigationController() //1. Declare an instance of UINavigationController
override func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?
) -> Bool {
GeneratedPluginRegistrant.register(with: self)
//2. Place the following 6 lines of code.Use these line if using the order version that 1.1.29
//let flutterViewController: FlutterViewController = window?.rootViewController as! FlutterViewController
//self.navigationController = UINavigationController(rootViewController: flutterViewController);
//self.navigationController.setNavigationBarHidden(true, animated: false)
//self.window = UIWindow(frame: UIScreen.main.bounds)
//self.window.rootViewController = self.navigationController
//self.window.makeKeyAndVisible()
return super.application(application, didFinishLaunchingWithOptions: launchOptions)
}
}
This completes the native Android and native iOS setup. Follow the next steps to proceed with the Flutter-specific setup of the plugin.
Flutter Setup
Register Package Name
Open your Flyy Dashboard (Staging) and go to Settings > Connect SDK and register a Package Name.
Note
- The package name need to be same as your Android app's application id if using the refer and earn.
- Package name once registered cannot be edited.
Set Package Name
Initialize the SDK from the project's main
method.
void main() async {
WidgetsFlutterBinding.ensureInitialized();
...
FlyyFlutterPlugin.setPackageName("<package_name>");
...
runApp(const MyApp());
}
Initialize SDK
The final step of initialization is to call the init
and the setUser
methods.
Initialize with a Partner ID
Right below the setPackageName
call, initialize the SDK. For integration purposes, set the environment as Flyy.STAGE
.
//For Production
FlyyFlutterPlugin.initFlyySDK("<partner_id>", FlyyFlutterPlugin.PRODUCTION);
//For Staging
FlyyFlutterPlugin.initFlyySDK("<partner_id>", FlyyFlutterPlugin.STAGE);
You can find the Partner ID in the Flyy Dashboard at Settings > SDK Keys.
Set User ID
To complete the initialization process, call the setUser
method. The setUser
method must be called every time a user opens the app.
//Set User ID. During Login and every subsequent app open.
FlyyFlutterPlugin.setFlyyUser("<unique_id>");
Note
unique_id can be any string that will be shown in reports. This must be unique and cannot be changed for any User later on.
Run the app.
To check whether the initialization was successful, open your Staging dashboard and go to Reports > Users. In case of successful integration, you should immediately see the newly created user in the Users Report. With that, the SDK Initialization is complete.
Add Flyy Widget (Optional)
The Flyy Widget provides a ready-to-use entry point leading to Flyy SDK's Primary Screens.
Adding the Flyy Widget is not required to complete the initialization process, but is recommended because it will allow you to test the SDK features readily.
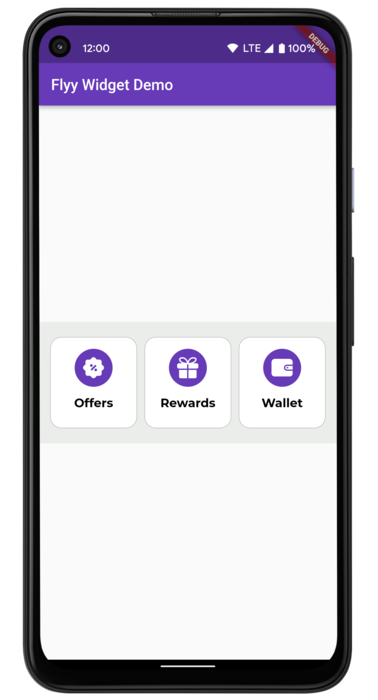
To add the Flyy Widget, just add the following widget to your layout.
FlyyFlutterPlugin.flyyWidget()
The Flyy Widget can also be customized to match your application's style and theme. To learn more, visit Flyy Widget's documentation page.
Manage Notifications
Flyy SDK takes advantage of Firebase's Cloud Messaging (FCM) to display Push Notifications and show in-app popups such as scratch cards. Therefore, it is necessary to set up FCM in your Flutter project. To set up FCM, follow its official guide.
Add FCM Server Key
To enable Flyy to send FCM notifications to your app perform either of the following steps in your dashboard.
Legacy HTTP API
- Copy the Server Key from Project Settings > Cloud Messaging in your Firebase console.
- Then paste it into your Flyy dashboard at Settings > SDK Keys.
HTTP v1 API
To use the newer API, the API requests must be authenticated using a Web API Key and a JSON Service Account Private Key. The below steps will guide you to get the API Key and JSON Key.
-
Provide API Key
-
Copy Web API Key from Project Settings > General in your Firebase console.
If this key is not yet generated, just perform the following two steps. Open Authentication and click Get Started. Go back to Project Settings > General to find the Web API Key generated. -
Paste the Web API Key in the API Key text box in your Flyy Dashboard at Settings > SDK Keys.
-
-
Upload JSON Key
- Generate new private key from Project Settings > Service accounts.
- Then, in your Flyy Dashboard, click Upload Service Account json file to upload the downloaded JSON file.
Click Save.
Send FCM Token to Flyy's Server
FCM Token should be sent to Flyy's Servers every time the FCM token is refreshed. Therefore, the following sendFCMTokenToServer call must be made during app startup.
Note, that this call is only required to be made from the iOS platform because on Android the SDK updates it automatically.
if (Platform.isIOS) {
FirebaseMessaging.instance.onTokenRefresh.listen((token) {
FlyyFlutterPlugin.sendFCMTokenToServer(token);
});
}
Redirect Flyy Notifications to the SDK
Handle Notification foreground Notifications (Android & iOS)
FirebaseMessaging.onMessage.listen((RemoteMessage remoteMessage) {
if (Platform.isAndroid) {
if (remoteMessage != null &&
remoteMessage.data != null &&
remoteMessage.data.containsKey("notification_source") &&
remoteMessage.data["notification_source"] != null &&
remoteMessage.data["notification_source"] == "flyy_sdk") {
FlyyFlutterPlugin.handleNotification(remoteMessage.data);
}
} else if (Platform.isIOS) {
if (remoteMessage.data.containsKey("notification_source") &&
remoteMessage.data["notification_source"] != null &&
remoteMessage.data["notification_source"] == "flyy_sdk") {
FlyyFlutterPlugin.handleForegroundNotification(remoteMessage.data);
}
}
}).onError((error) {
print(error);
});
Handle Background Notification For iOS
void main() async {
...
// Call from the main method
FirebaseMessaging.onBackgroundMessage(_firebaseMessagingBackgroundHandler);
runApp(const MyApp());
}
/// It must be a top-level function (e.g. not a class method which requires initialization).
Future<void> _firebaseMessagingBackgroundHandler(
RemoteMessage remoteMessage) async {
if (remoteMessage.data.containsKey("notification_source") &&
remoteMessage.data["notification_source"] != null &&
remoteMessage.data["notification_source"] == "flyy_sdk") {
FlyyFlutterPlugin.handleBackgroundNotification(remoteMessage.data);
}
}
Prepare for Release
If you are using ProGuard to obfuscate your code, then add the following rules to your proguard-rules.pro file (your_project/android/proguard-rules.pro):
#Retrofit does reflection on generic parameters. InnerClasses is required to use Signatureand
# EnclosingMethod is required to use InnerClasses.
-keepattributes Signature, InnerClasses, EnclosingMethod
# Retrofit does reflection on method and parameter annotations.
-keepattributes RuntimeVisibleAnnotations, RuntimeVisibleParameterAnnotations
#Retain service method parameters when optimizing.
-keepclassmembers,allowshrinking,allowobfuscation interface * { @retrofit2.http.* <methods>;}
# Ignore annotation used for build tooling.
-dontwarn org.codehaus.mojo.animal_sniffer.IgnoreJRERequirement
# Ignore JSR 305 annotations for embedding nullability information.
-dontwarn javax.annotation.**
# Guarded by a NoClassDefFoundError try/catch and only used when on the classpath.
-dontwarn kotlin.Unit
# Top-level functions that can only be used by Kotlin.
-dontwarn retrofit2.KotlinExtensions
-dontwarn retrofit2.KotlinExtensions$*
# With R8 full mode, it sees no subtypes of Retrofit interfaces since they arecreated withaProxy
# and replaces all potential values with null. Explicitly keeping the interfacesprevents this.
-if interface * { @retrofit2.http.* <methods>; }
-keep,allowobfuscation interface <1>
-keep class retrofit2.** { *; }
-keep public class theflyy.com.flyy.** {*;}
Updated 4 months ago