Create your First Campaign
Create and implement your first campaign in your iOS
This guide is aimed to help onboard iOS Developers who are new to using the Flyy SDK. You'll be learning how to:
- Create a new Campaign from the Flyy dashboard. The goal of this campaign will be to reward users whenever they make a purchase.
- Implement the offer in an iOS app to reward users when they tap the Checkout Button.
- View how the app performs from a user's perspective.
- Track claimed offers from Reports.
Prerequisites
- It is required that you've already followed and completed all the steps from the Setup Guide.
Create a Campaign from the Dashboard
Set up a Challenges campaign from your staging dashboard by following these instructions. When done, come back and implement this offer in the ios app.
Implement Offer in the iOS App
Download the demo project for the sample app from this GitHub repository. The demo project just contains the basic setup and an extra view to simplify this guide. Before we can run this code, we need to configure the project to connect it with the Flyy dashboard as well as your Firebase project.
Configure the Demo Project
Config Step 1: Set Package Name
Double click on the file FlyyX Integration.xcworkspace to open the project in Xcode.
In the AppDelegate.swift
, provide the Package Name copied from your Flyy dashboard at Settings > Connect SDK
func application(_ application: UIApplication, didFinishLaunchingWithOptions
launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
...
//TODO: Config 1: Set Package Name
Flyy.sharedInstance.setPackage(packageName:"")
...
}
Flyy *flyy;
@implementation AppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
//Initialize flyy variable
flyy = [Flyy sharedFlyyInstance];
//TODO: Config 1: Set Package Name
[flyy setPackageWithPackageName:@"{package_name"];
...
return YES;
}
Config Step 2: Set Partner ID
Copy Partner ID from your Flyy dashboard at Settings > SDK Keys and paste it in the init
method.
//TODO: Config 2: Paste Partner Id from "Settings > SDK Keys" in Dashboard.
Flyy.sharedInstance.initSDK(partnerToken: "partner_id", environment: Flyy.FLYY_ENVIRONMENT_STAGING)
//TODO: Config 2: Paste Partner Id from "Settings > SDK Keys" in Dashboard.
[flyy initSDKWithPartnerToken:@"{partner_id}" environment: [flyy staging]];
Config Step 3: Download GoogleService-Info.plist
From the same Firebase Project used to setup Firebase Messaging to Manage Notification, download the GoogleService-Info.plist file from your project's General Settings and place at the root of your Xcode project. This plist file must be added using Xcode for it to generate a reference.
Config Step 4: Add a Bundle Identifier
Add a Bundle Identifier for the project from Xcode. Click on the project under TARGETS and go to Signing & Capabilities and the add Bundle Identifier. The Bundle Id should match the one mentioned in the GoogleService-info.plist file.
Config Step 5: Install pod dependency
Open Terminal at the root of this project's directory and run pod install
to install FlyyFramework dependency.
Reopen the project by double-clicking FlyyX Integration.xcworkspace and Run the app.
Go to Reports > Users in your Flyy Dashboard. You should see a new user test_user_ios.
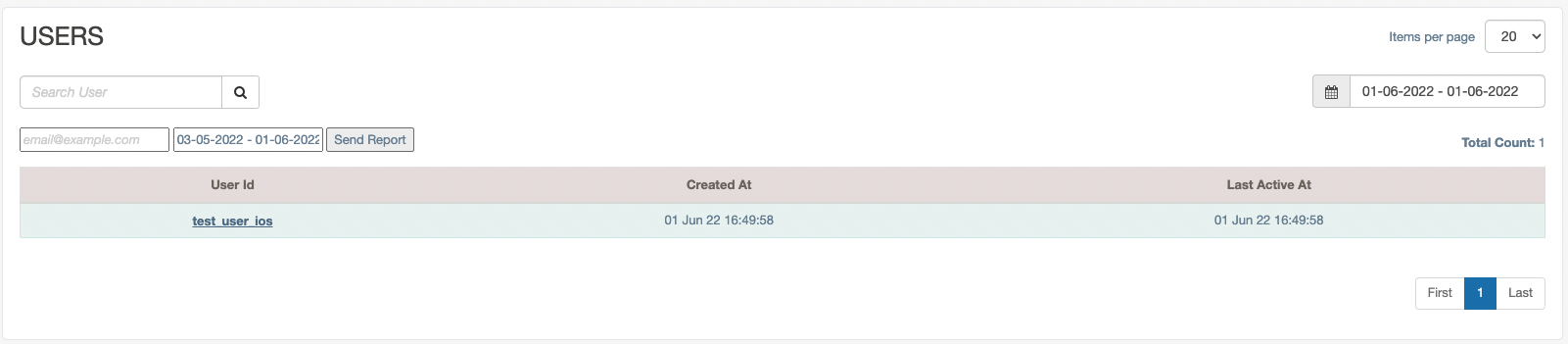
View Offers
On the Offers Page user can see all the Offers currently available to them. With Flyy's Plug and Play capability, we can navigate to the Offers Page by just adding a single line of code.
In the ViewController.swift, locate the openOffers
method and add the following line of code.
@IBAction func openOffers(_ sender: Any) {
//TODO: 1. Navigate to Offers Page
Flyy.sharedInstance.openOffersPage()
}
- (IBAction)openOffers:(id)sender {
//TODO: 1. Navigate to Offers Page
[flyy openOffersPage];
}
Run App and Tap on the Offers button to view the following screen.
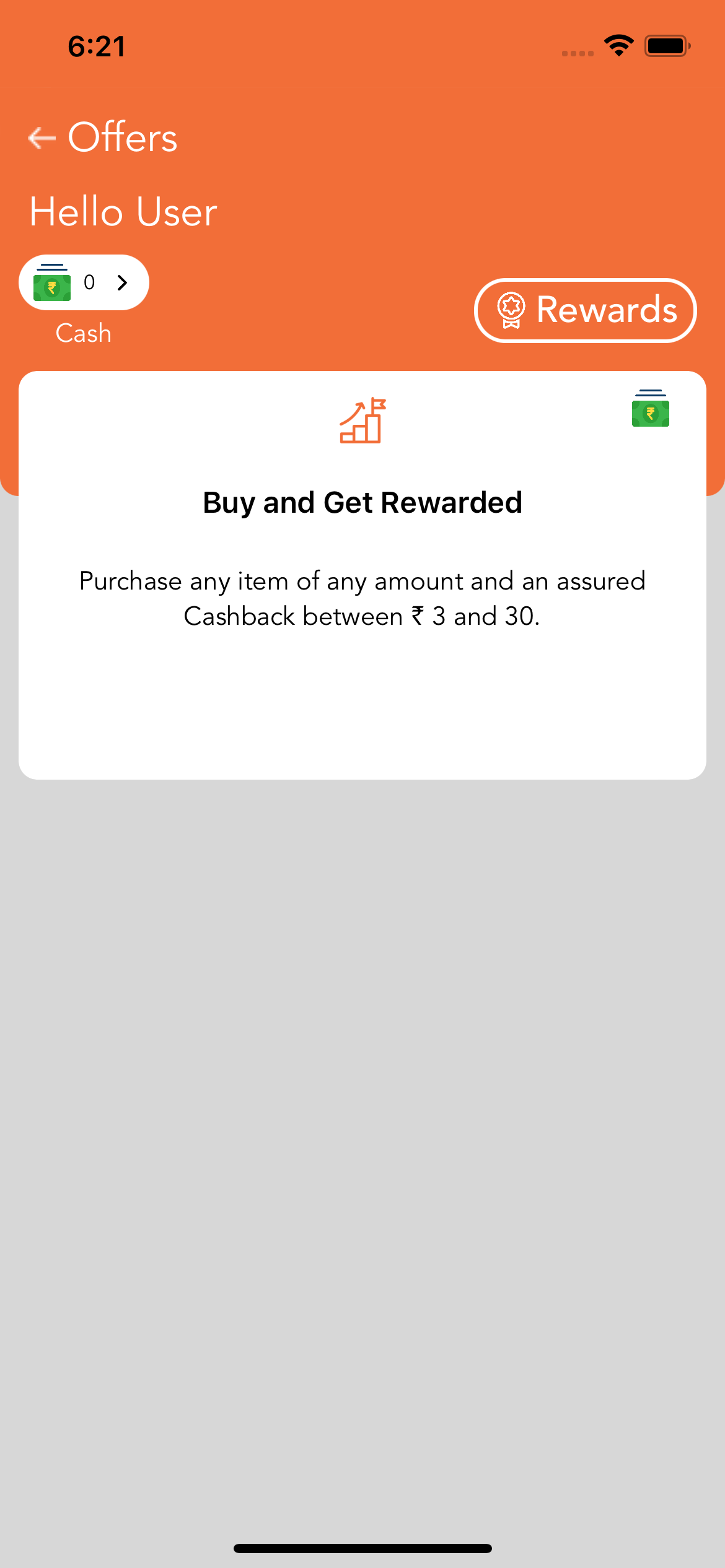
Tap on Back to return to the Home Screen.
Send Event on Checkout
There is just one final step remaining and that is sending the purchase event at Checkout.
In Xcode, open CartViewController.swift and add the following line to the checkout
method.
@IBAction func checkout(_ sender: Any) {
//TODO: 2. Send purchase Event
Flyy.sharedInstance.sendEvent(key: "purchase", value: "true")
}
- (IBAction)checkout:(id)sender {
//TODO: 2: Send purchase Event
[flyy sendEventWithKey:@"purchase" value:@"true"];
}
The sendEvent
method takes in two arguments: the first one is the Event Key, and the second is a JSON object to send conditions in key-value pairs.
Since we don't have any conditions, the second argument is just a placeholder and can be any text besides "true"
as well.
Sending User Event with API
The
sendEvent
method should only be used for testing on the Staging environment.
For production, use the Sending User Event API call from your backend instead. AllsendEvent
events generating from the front-end are ignored by Flyy's reward system.
Now, let's Run the App one more time and perform the following actions.
Open Cart Page and tap Checkout. A popup along with a push notification will appear on the screen after a moment.
Track Campaign
View Event Status
Back in the Flyy Dashboard, go to Settings > Events. the Connection Status for the purchase event should now be Connected.
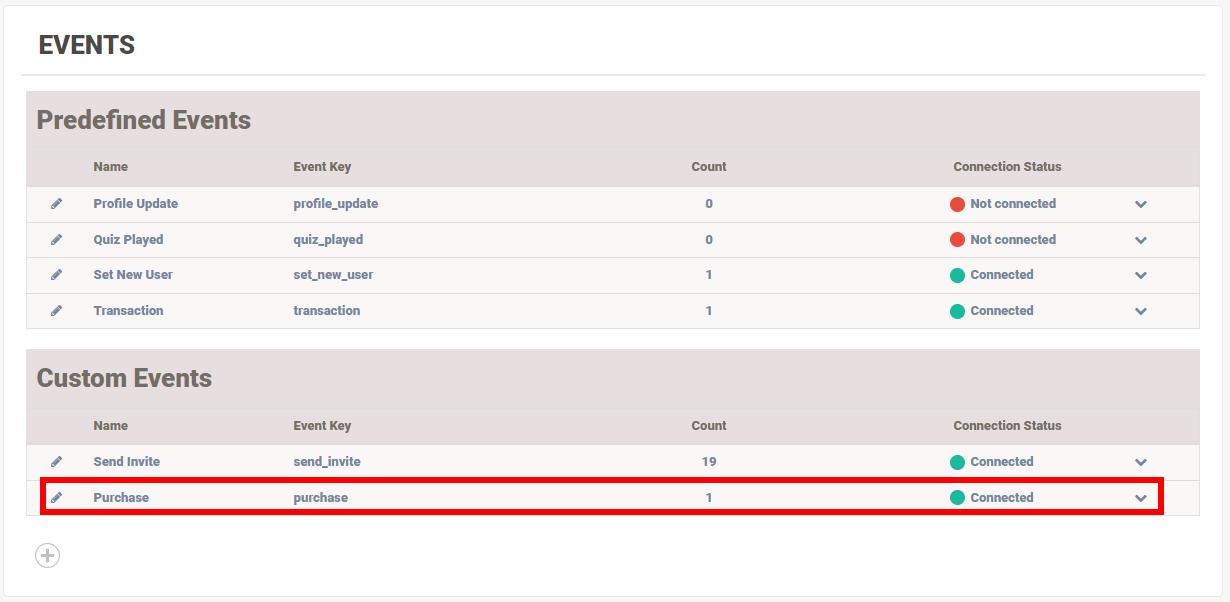
Updated about 3 years ago