SDK Documentation
Overview of all the SDK features available
Primary Screens
Offers Screen
To open the offers screen for the user.
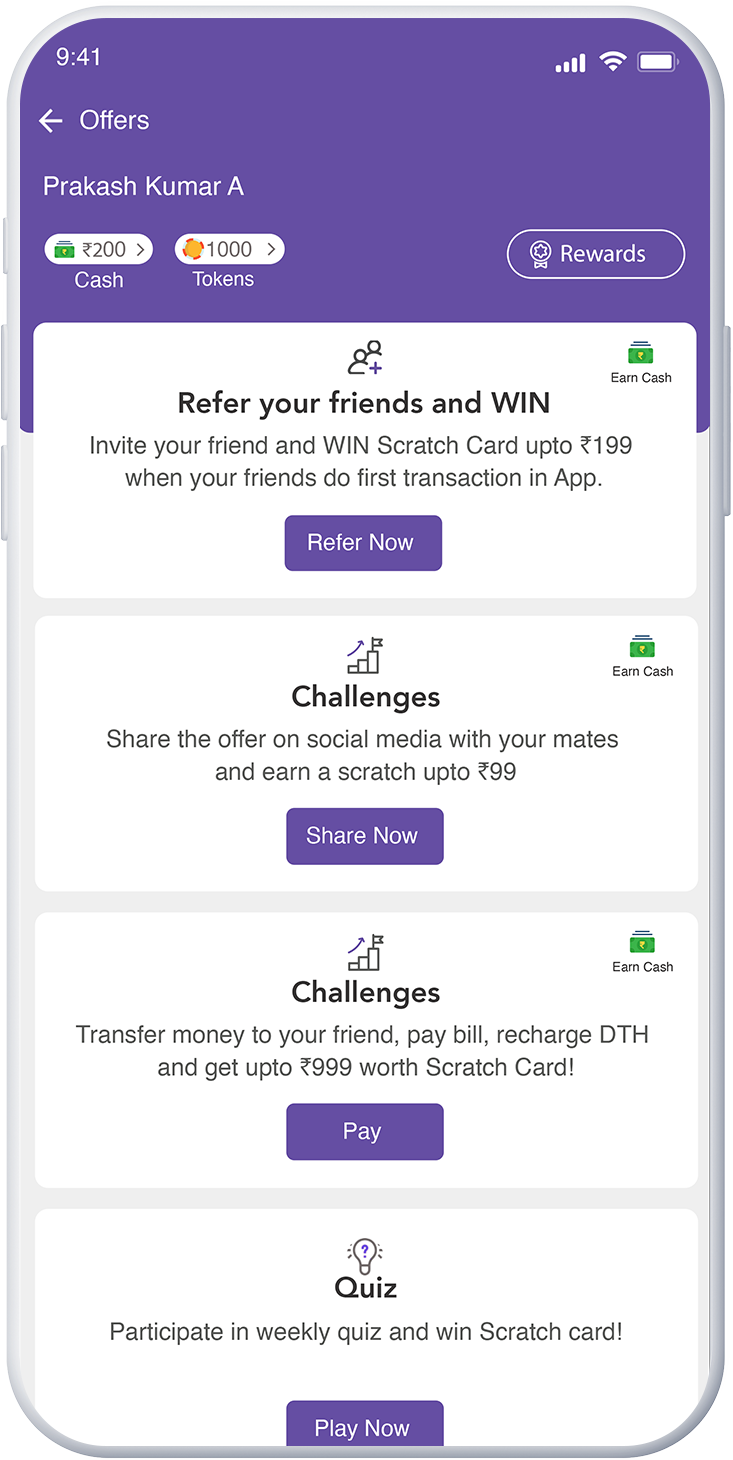
Flyy.openOffersScreen();
Rewards Screen
To open rewards screen for user.
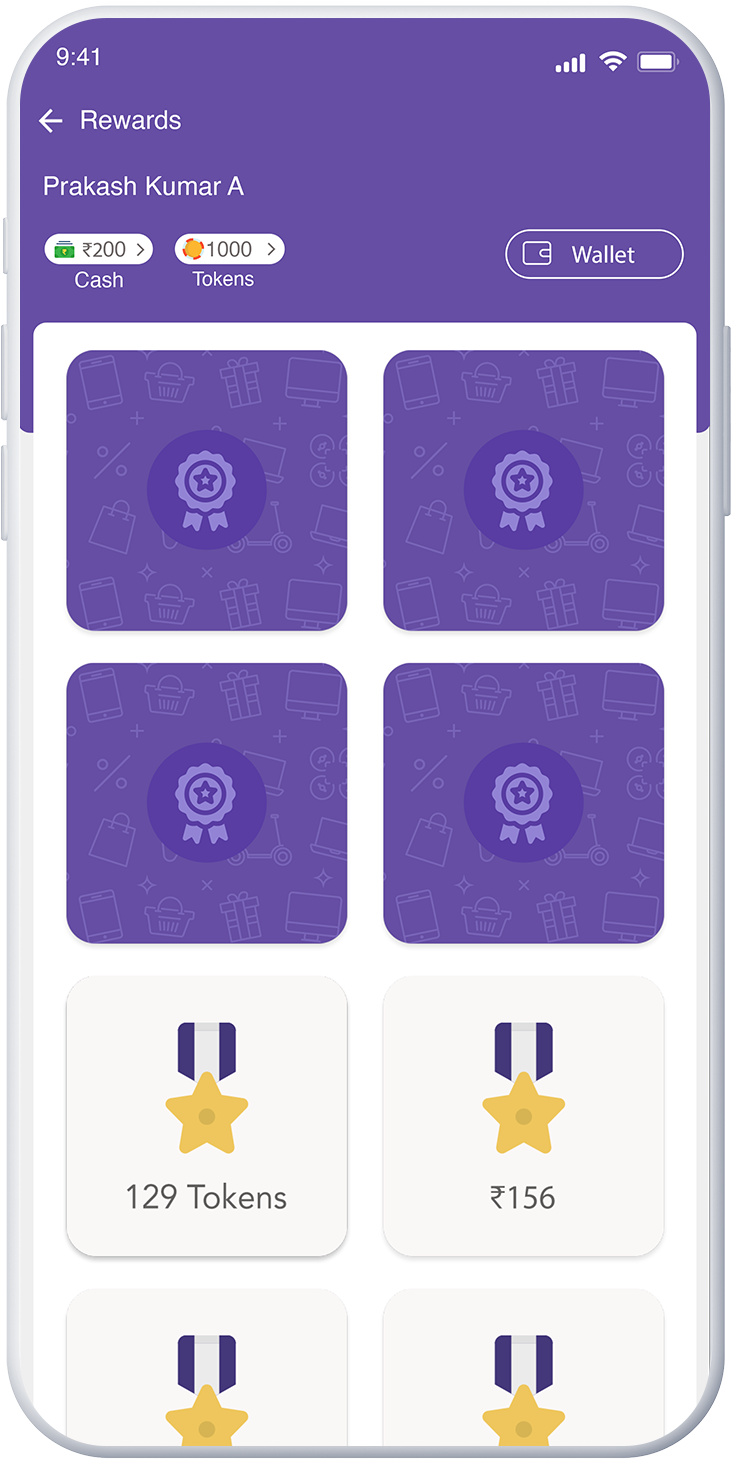
Flyy.openRewardsScreen();
Wallet Screen
To open wallet screen for user
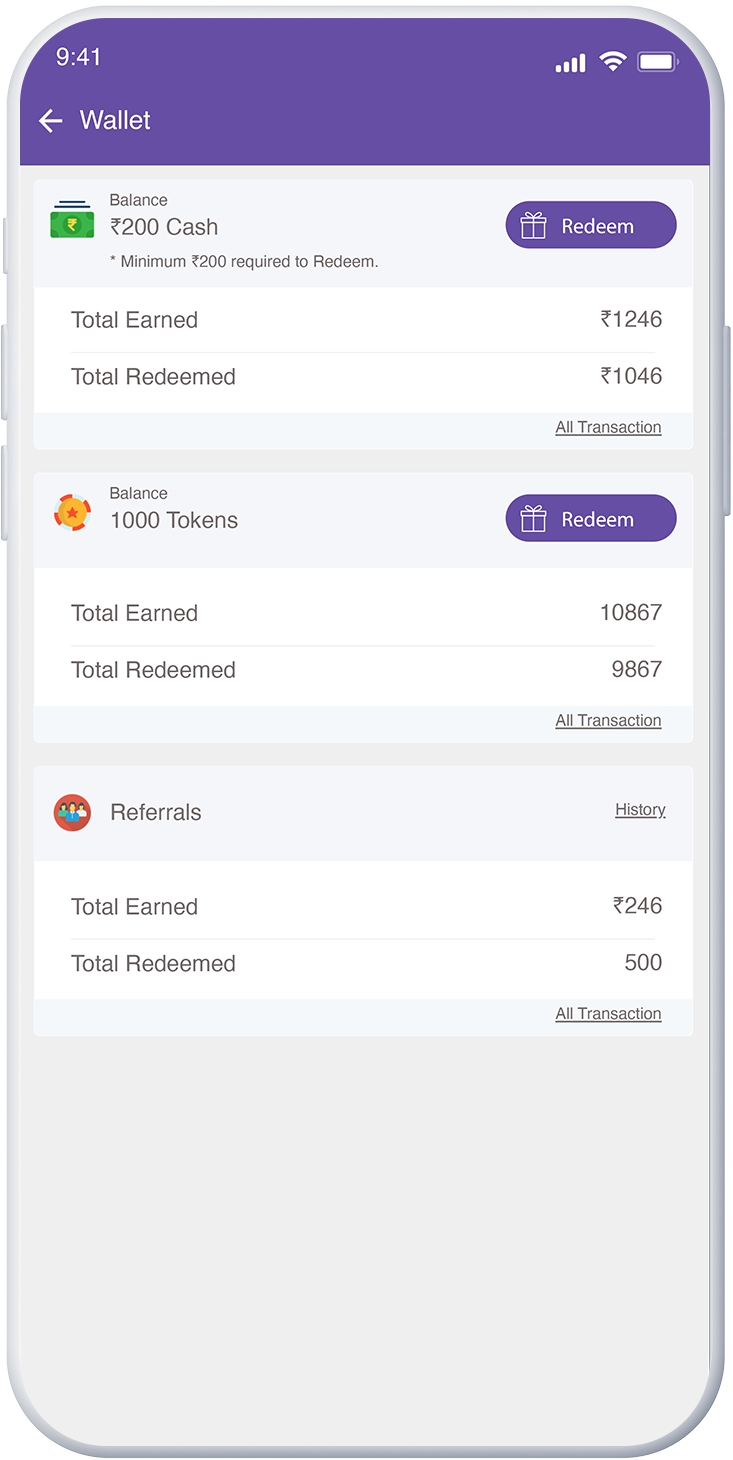
Flyy.openWalletScreen();
Additional Screens
Gift Cards Screen
To open the gift cards screen for the user
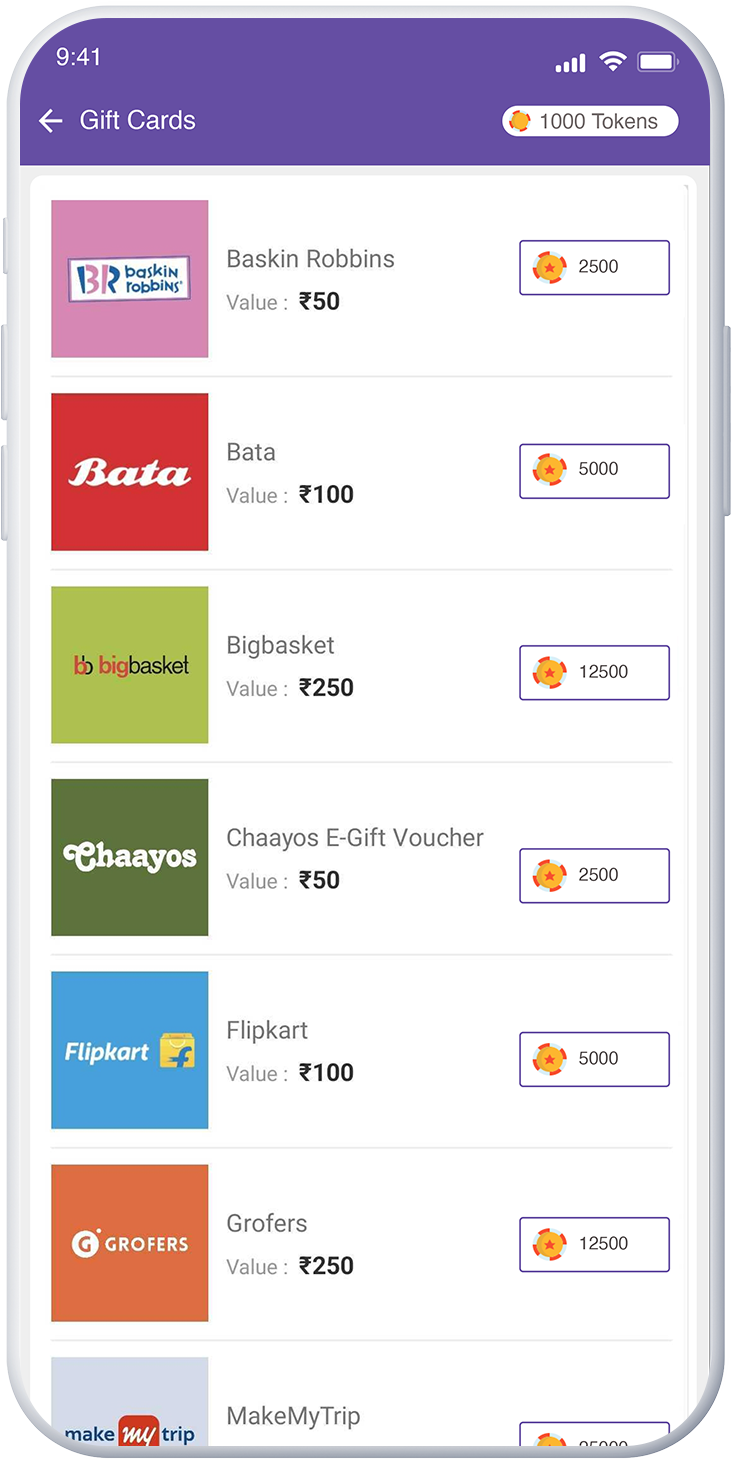
Flyy.openGiftCardScreen();
Referral History Screen
To open the referral history screen for the user
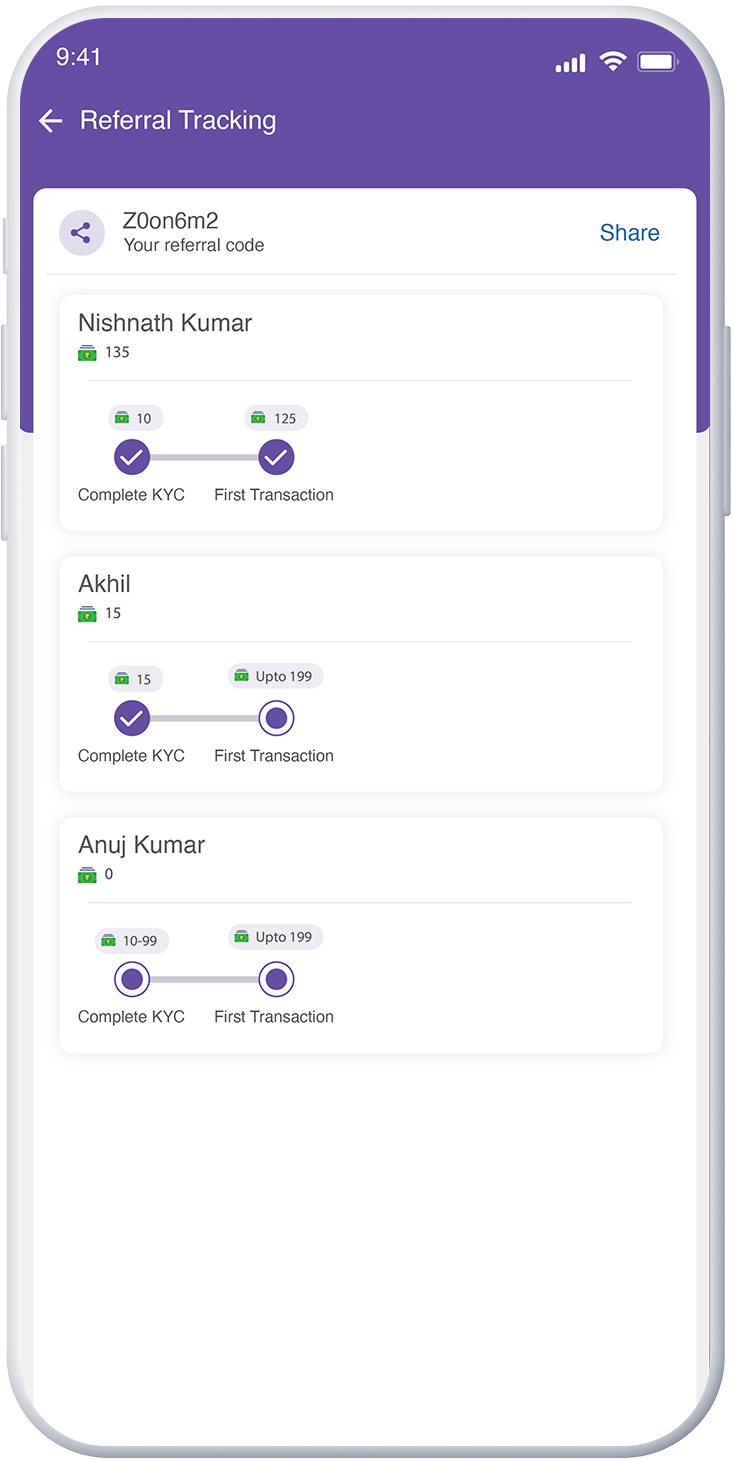
Flyy.openReferralHistory();
Quiz Screen
To open quiz screen for the user
Flyy.openFlyyQuizPage(0);
//live quiz_id or 0
Quiz History Screen
To open the quiz history screen for the user
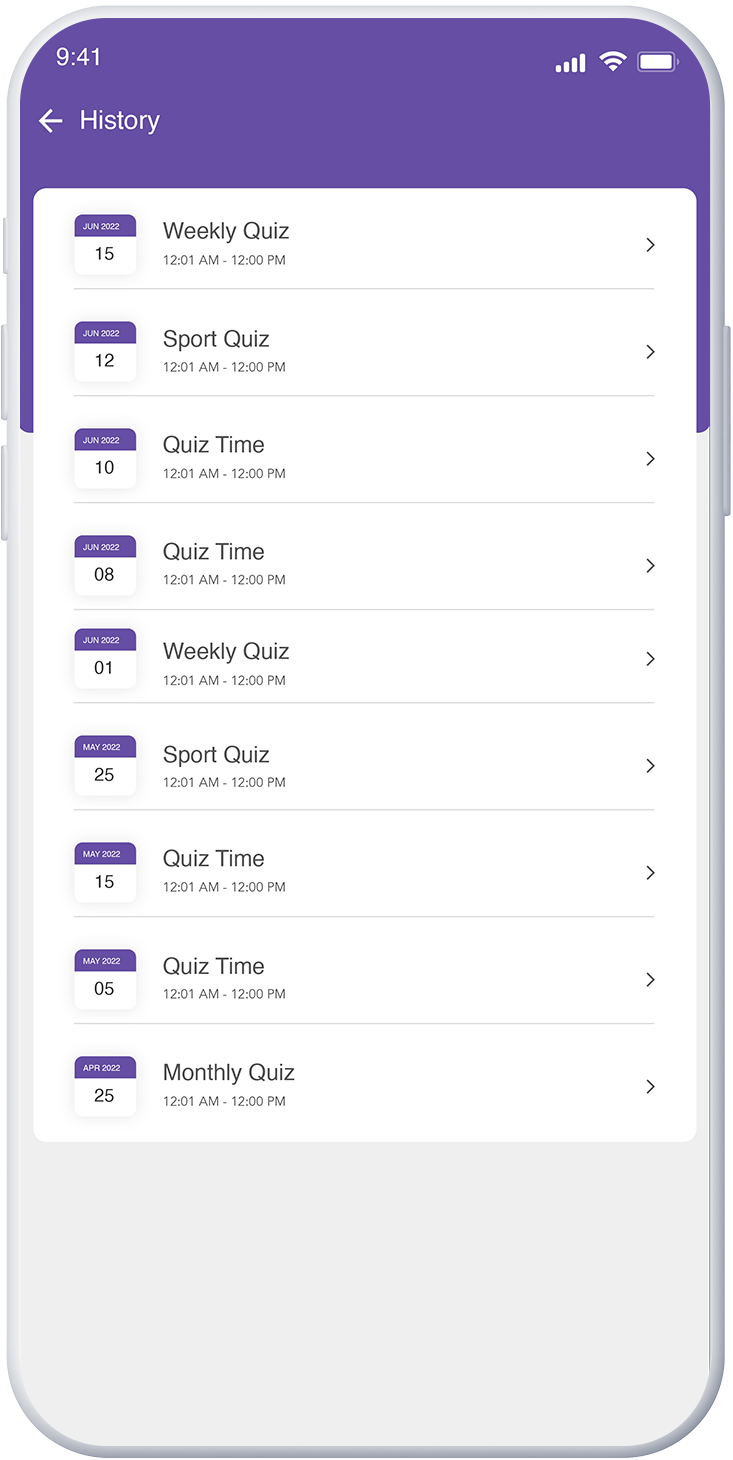
Flyy.openFlyyQuizHistoryPage();
Quiz List Screen
To open the quiz list screen for the user.
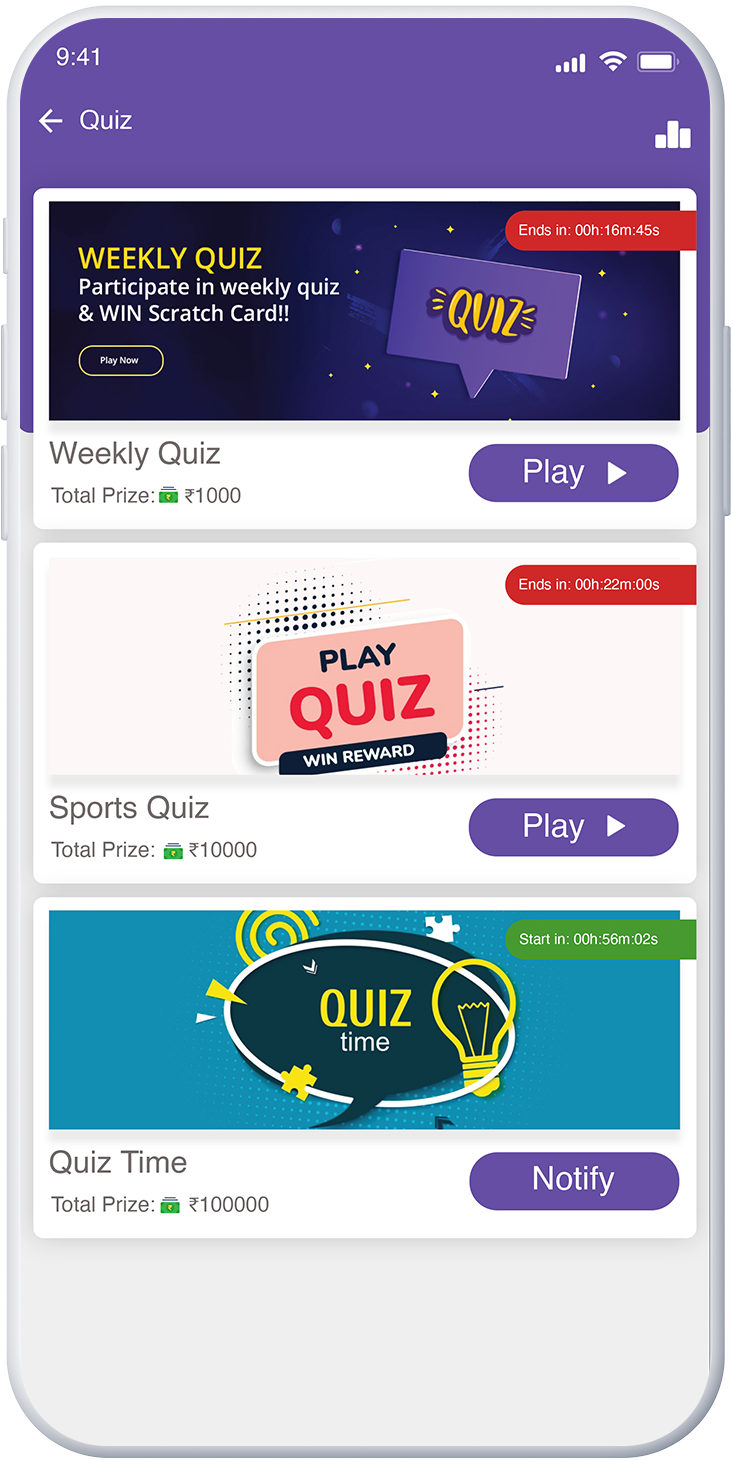
Flyy.openFlyyQuizListPage();
Stamps Screen
To open the stamps screen for the user.
Flyy.openFlyyStampsPage();
Invite Detail Screen
To open the invite detail screen for the user.
Flyy.openFlyyInviteDetailsPage(2);
//offer_id can be null
Invite And Earn Screen
To open the invite and earn screen for the user.
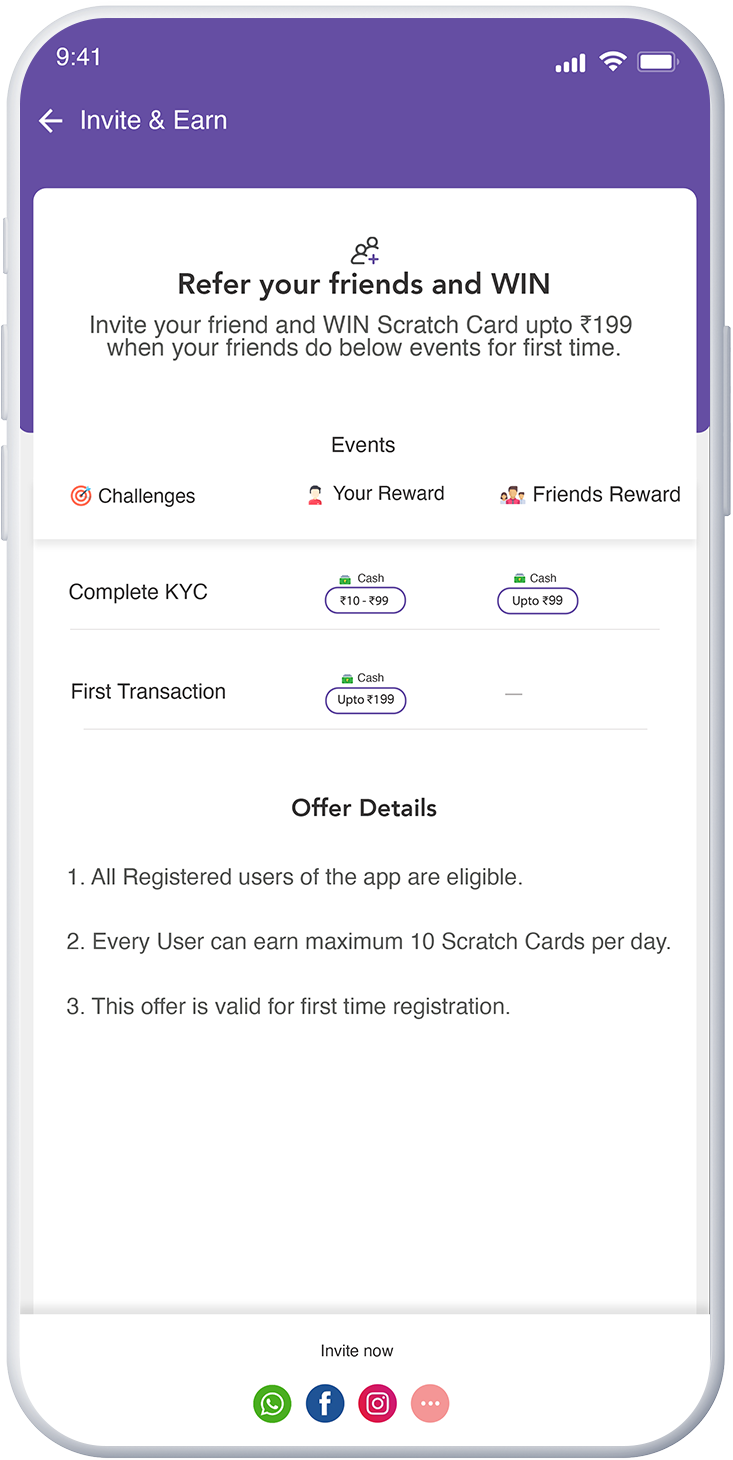
Flyy.openFlyyInviteAndEarnPage(2);
//offer_id can be null
Custom Invite and Earn Screen
To open a custom invite and earn screen for the user.
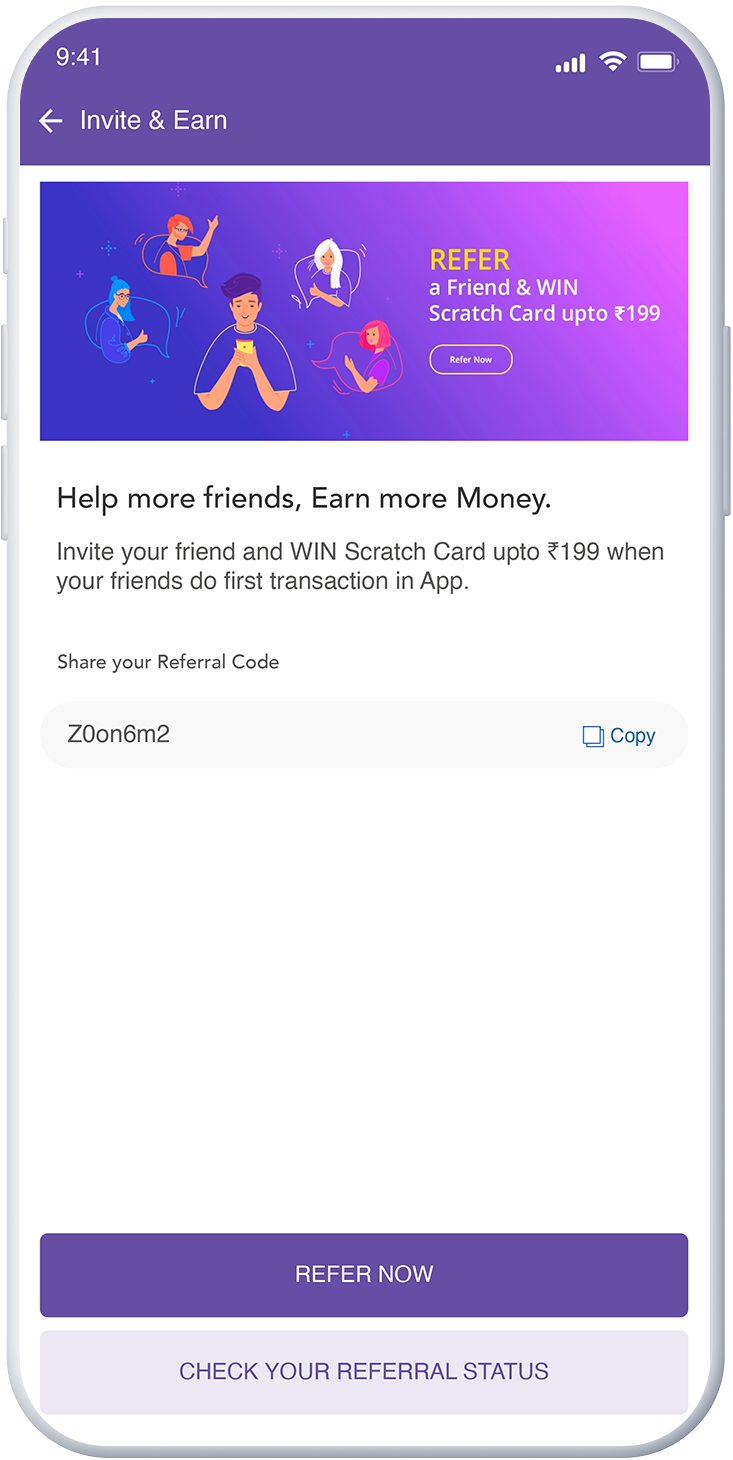
Flyy.openFlyyCustomInviteAndEarnPage(2, “#ffffff”);
//offer_id can be null
//tool bar items default color will be black if color is not passed
Challenge Details Screen
To open the challenge details screen for the user.
Flyy.openFlyyChallengeDetailPage(2);
//offer_id can be null
Bonanza Screen
To open a bonanza details screen for user.
Flyy.openFlyyBonanzaPage();
Additional Methods
Set Theme Colors
The Flyy SDK inherits theme colors which are provided to the setThemeColor method. The setThemeColor method must be called immediately after the init method is called.
//Parameters to be passed are colorPrimary and colorPrimaryDark
Flyy.setThemeColor("#FF5733", "#842C19");
Set Name
This will be shown in the screens wherever username is displayed.
Flyy.setUserName("<user_name>");
SDK Initialization with referral callback
Initialize sdk in your application or main entry point of the app with referral callback as shown below.
//For Production
Flyy.initSDKWithReferralCallback("<partner_id>", Flyy.PRODUCTION,
(referralData) => {
});
//For Staging
Flyy.initSDKWithReferralCallback("<partner_id>", Flyy.STAGE,
(referralData) => {
});
Set User Token
To increase security for your users you can set unique user token in the following manner instead of setUser() method
///without callback
Flyy.setUserToken("BSt4Y3A2"); //pass your user token
Set User Token along with Segment ID
To increase security for your users you can set unique user token along with segment id in the following manner instead of setUser() method
//without callback
Flyy.setUserTokenWithSegment("BSt4Y3A2", "all_users");
Set New User Token
To increase security for your users you can set unique new user token for new registered users in the following manner instead of setNewUser() method
Flyy.setNewUserToken("QWt4Y3A2"); //pass your new user token
Set New User Token along with Segment ID
To increase security for your users you can set unique new user token for new registered users along with segment id in the following manner instead of setNewUser() method
//without callback
Flyy.setNewUserTokenWithSegment("QWt4Y3A2", "all_users");
Set Bank Details
You can use the following method to set user redemption details, which takes following params,
- Account number - bank account number
- IFSC code
- Name
Flyy.setBankDetails("0000xxxxxxxxxx16", "BSxxxxxxx0", "Pooja");
Set UPI Details
You can use the following method to set user redemption details, which takes following params,
- UPI ID
Flyy.setUPIDetails("user@upiId");
Send Events
Values can either be sent in the form of key and value or as a json object.
Flyy.sendEvent("profile_updated", "true");
//OR
Flyy.sendEvent("registration", <JSON.stringify(data)>);
Set Referral Code
To set referral code, please call the following function
Flyy.setReferralCode("sdfhyr6");
Get Referral Code
To get referral code, please call the following function
Flyy.getReferralCode((referralCode) => {
console.log(referralCode);
});
Verify Referral Code
To verify if referral code is valid or not, please call the following function
Flyy.verifyReferralCode("sdfh123",
(isValid, referralCode) => {
console.log(isValid, referralCode);
});
Get Share Data
Use the below code to get share data. In the callback you will receive following params
- referral_link - Referral link to share as string
- referral_message - Referral message to share as string
- share_message - Share message as string
Flyy.getShareData(
(referralDetails) => {
console.log("Referral Link " + referralDetails.referral_link);
console.log("Referral Message " + referralDetails.referral_message);
console.log("Share Message" + referralDetails.share_message);
});
Get Referral Count
Use the below code to get referral count. In the callback you will receive referral count as integer.
Flyy.getReferralCount(
(referralCount) => {
console.log("Referral Count " + referralCount);
});
Get Scratch Card Count
Use the below code to get scratch card count.
Flyy.getScratchCardCount(
(scratchCardCountData) => {
console.log("Scratched SC " + scratchCardCountData.scratched_sc_count);
console.log("UnScratched SC " + scratchCardCountData.unscratched_sc_count);
console.log("Total SC " + scratchCardCountData.total_sc_count);
console.log("Locked SC " + scratchCardCountData.locked_sc_count);
});
Get Previous Leaderboard Winner
Use the below code to get previous leaderboard winners.
Flyy.getPreviousLeaderboardWinners("test",
(previousLeaderBoardWinners) => {
console.log("Participants count " + previousLeaderBoardWinners.participants_count);
console.log("Winners data " + JSON.stringify(previousLeaderBoardWinners.winners));
console.log("Previous Winners data " + JSON.stringify(previousLeaderBoardWinners.previous_winners));
}
);
Get Leaderboard Participants
Use the below code to get leaderboard participants.
Flyy.getLeaderboardParticipants("test",
(leaderBoardWinners) => {
console.log("Participants count " + leaderBoardWinners.participants_count);
console.log("Winners data " + JSON.stringify(leaderBoardWinners.winners));
console.log("Previous Winners data " + JSON.stringify(leaderBoardWinners.previous_winners));
}
);
Get Wallet Balance
Use the below code to get wallet balance.
Flyy.getWalletBalance("cash",
(walletBalanceData) => {
console.log("Balance " + walletBalanceData.balance);
console.log("Total Credit " + walletBalanceData.total_credit);
console.log("Total Debit " + walletBalanceData.total_debit);
});
Get Referrer Details
Use the below code to get referrer details.
Flyy.getReferrerDetails(
(referralData) => {
console.log("Name " + referralData.name);
console.log("Ext UID " + referralData.ext_uid);
console.log("Extra Data " + referralData.extra_data);
});
Get Offers Count
Use the below code to get offers count.
Flyy.getOffersCount(
(offersCountData) => {
console.log("Live offers count " + offersCountData.live_offers_count);
console.log("Participated offers count " + offersCountData.participated_offers_count);
});
Updated almost 3 years ago