Create your First Campaign
Create and Implement your First Campaign in your Flutter Project
This guide is aimed at onboarding Flutter Developers who are new to using the Flyy SDK. You'll be learning how to:
- Create a new Campaign from the Flyy dashboard. The goal of this campaign will be to reward users whenever they make a purchase.
- Implement the offer in a Flutter project to reward users when they tap the Checkout Button.
- View how the app performs from a user's perspective.
- Track claimed offers by viewing Reports.
Prerequisites
- It is required that you've already followed and completed all steps in the Setup Guide.
Create a Campaign from the Dashboard
Set up a Challenges campaign from your staging dashboard by following these instructions. When done, come back and implement this offer in the Flutter project.
Implement Offer in a Flutter Project
Download the demo project for the sample app from this GitHub repository. The demo project just contains the basic setup and an extra activity to simplify this guide. Before we can run this code, we need to configure the project to connect it with the Flyy dashboard as well as your Firebase project.
Configure the Demo Project
Config Step 1: Set Package Name
In main.dart
, provide the Package Name copied from your Flyy dashboard at Settings > Connect SDK
void main() async {
...
//TODO Config 1: Paste Package name from "Settings > Connect SDK" in Dashboard.
FlyyFlutterPlugin.setPackageName("package_name");
...
runApp(const MyApp());
}
Config Step 2: Set Partner ID
Copy Partner ID from your Flyy dashboard at Settings > SDK Keys and paste it in the initFlyySDK
method.
//TODO Config 2: Paste Partner Id from "Settings > SDK Keys" in Dashboard.
FlyyFlutterPlugin.initFlyySDK("partner_id", FlyyFlutterPlugin.STAGE);
Config Step 3: Edit Package Name
Provide an Application Id for the Android Project and a Bundle Identifier for the iOS project. These should be the same as the ones used in the Firebase Project.
Follow the links for instructions on how to edit the Application Id on Android and Bundle Identifier on iOS.
Config Step 4: Configure Firebase
Note
Use the same Firebase project used for setting up FCM to Firebase Notification.
Flutter 2.8+
With the release of Flutter 2.8, Firebase can be configured for the project using the Firebase CLI.
Run the following command from the project's root directory and follow the steps presented in the terminal window.
flutterfire configure
Performing this step automatically downloads the required configuration files for both iOS and Android projects.
Pre Flutter 2.8
For projects using Flutter versions older than 2.8, should manually download the json and plist files and place them under relevant directories.
For instructions, follow the instructions provided at the link: Android, iOS.
Run App.
Go to Reports > Users in your Flyy Dashboard. You should see a new user test_user_1.
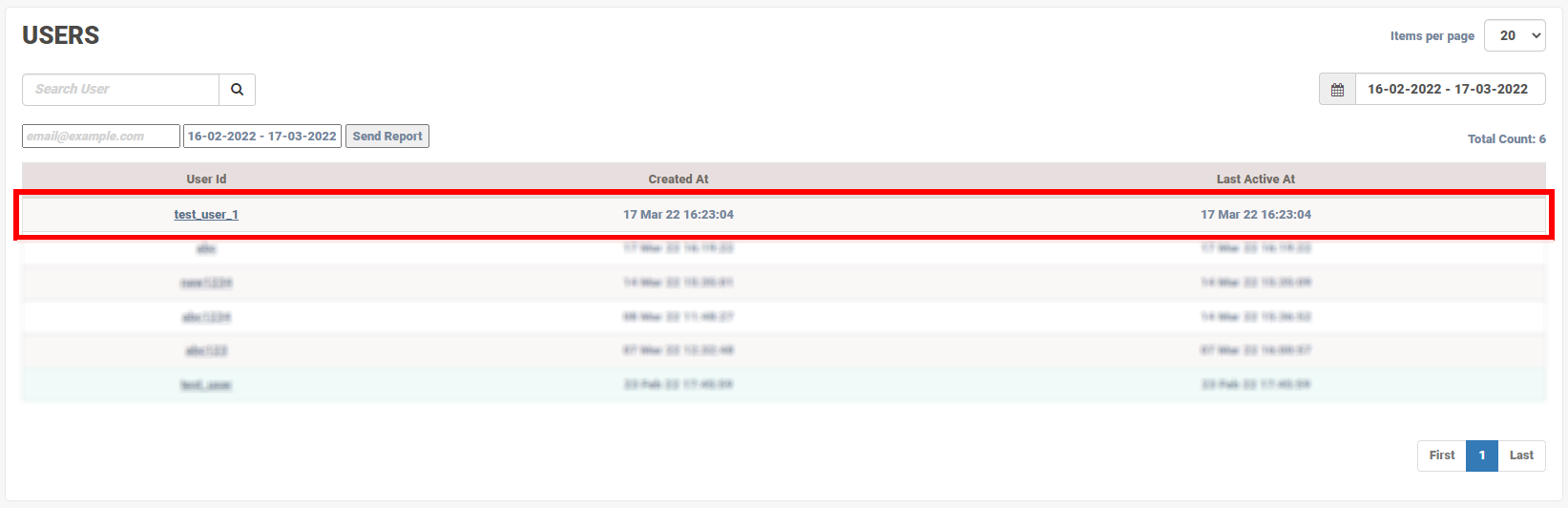
View Offers
The Offers page is where a user can see all the Offers currently available to them. Let us view what the user sees when they open the Offers Page.
Within the onPressed
property of the Offers button in main.dart, add the following line to open the Offers Page.
ElevatedButton(
onPressed: () {
//TODO Step 1: Navigate to Offers Page
FlyyFlutterPlugin.openFlyyOffersPage();
},
child: const Text("Offers"),
),
Run App and Tap on the Offers button to view the following screen.
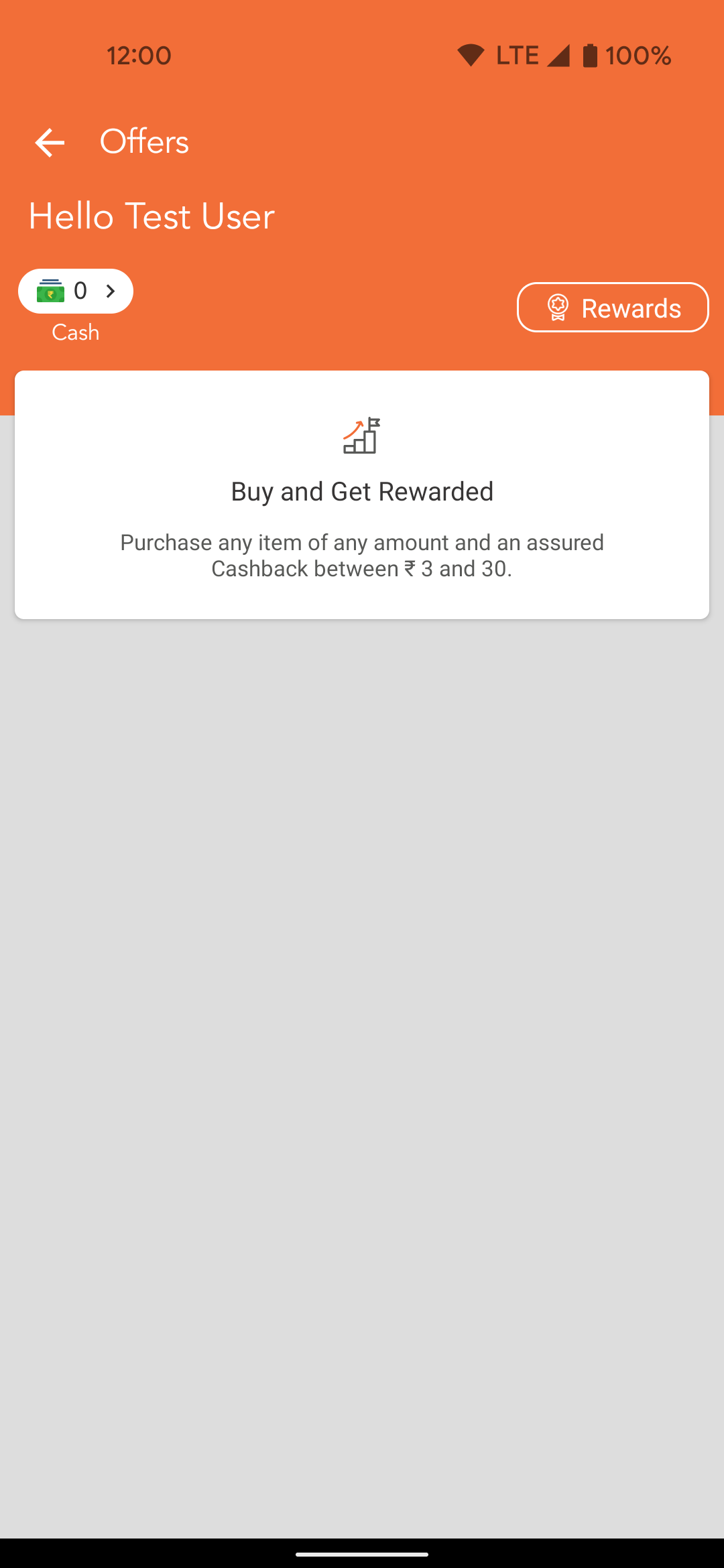
Flyy provides multiple ways to customize the look and feel of every campaign. Enabling Show Banner under Content & Styling will replace the above title and description with the provided banner image.
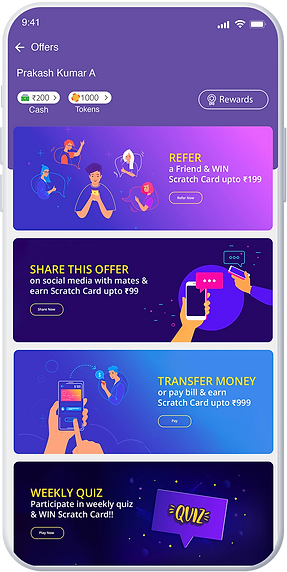
Navigate back to the previous screen.
Send Event on Checkout
There is just one final step remaining: sending the purchase event at Checkout.
In the IDE, open cart_screen.dart and add the following line to the Checkout button's onPressed
property.
ElevatedButton(
onPressed: () {
//TODO Step 2: Send purchase Event
FlyyFlutterPlugin.sendEvent("purchase", "true");
},
child: const Text("Checkout"),
),
The sendEvent
method takes in two arguments: the first one is the Event Key, and the second is a String to send conditions in key-value pairs.
Since we don't have any conditions, the second argument is just a placeholder and can be any text besides "true"
as well.
Sending User Event with API
The
sendEvent
method should only be used for testing on the Staging environment.
For production, use the Sending User Event API call from your backend instead. AllsendEvent
events generating from the front-end are ignored by Flyy's reward system.
Now, run the app and perform the following actions.
Open the Cart Page and tap Checkout. A popup and a push notification will appear on the screen after a moment.
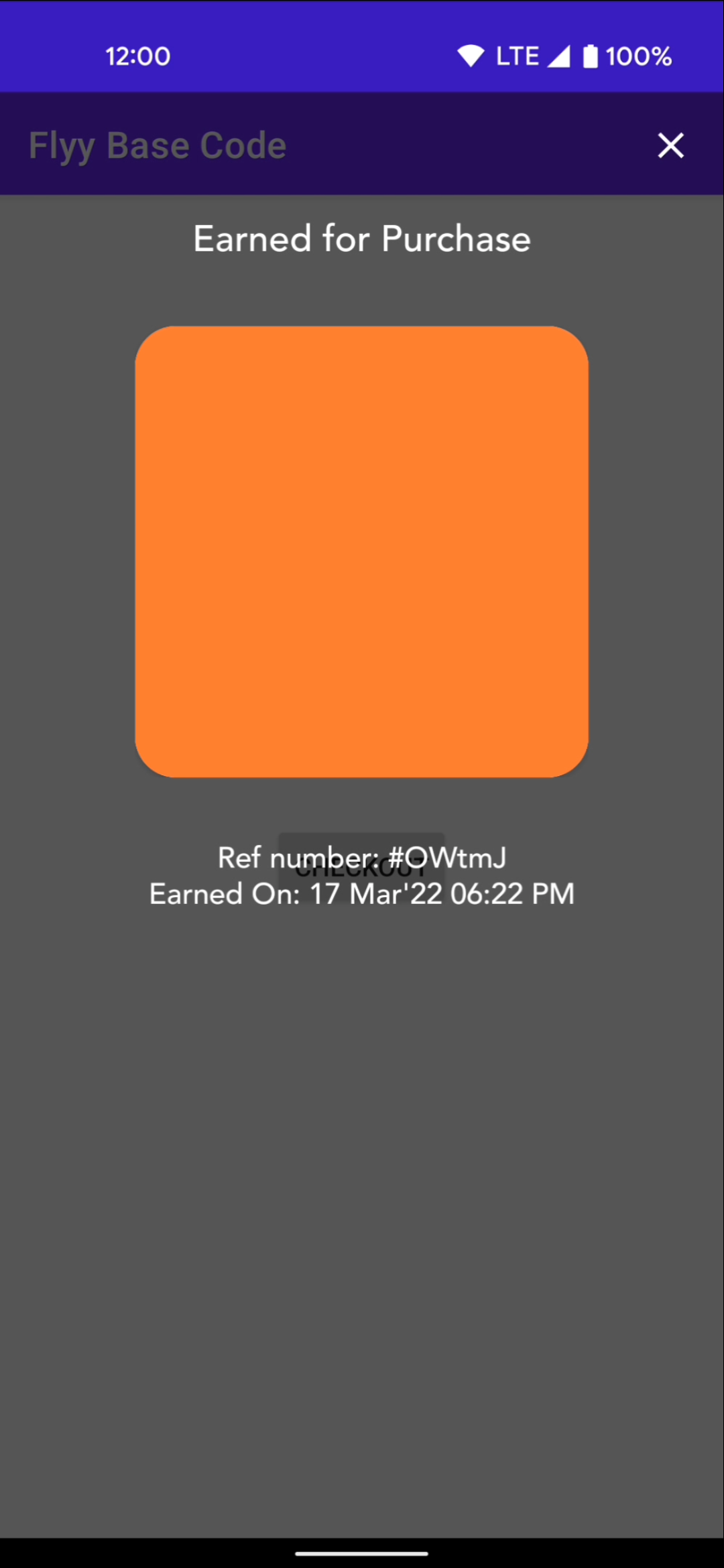
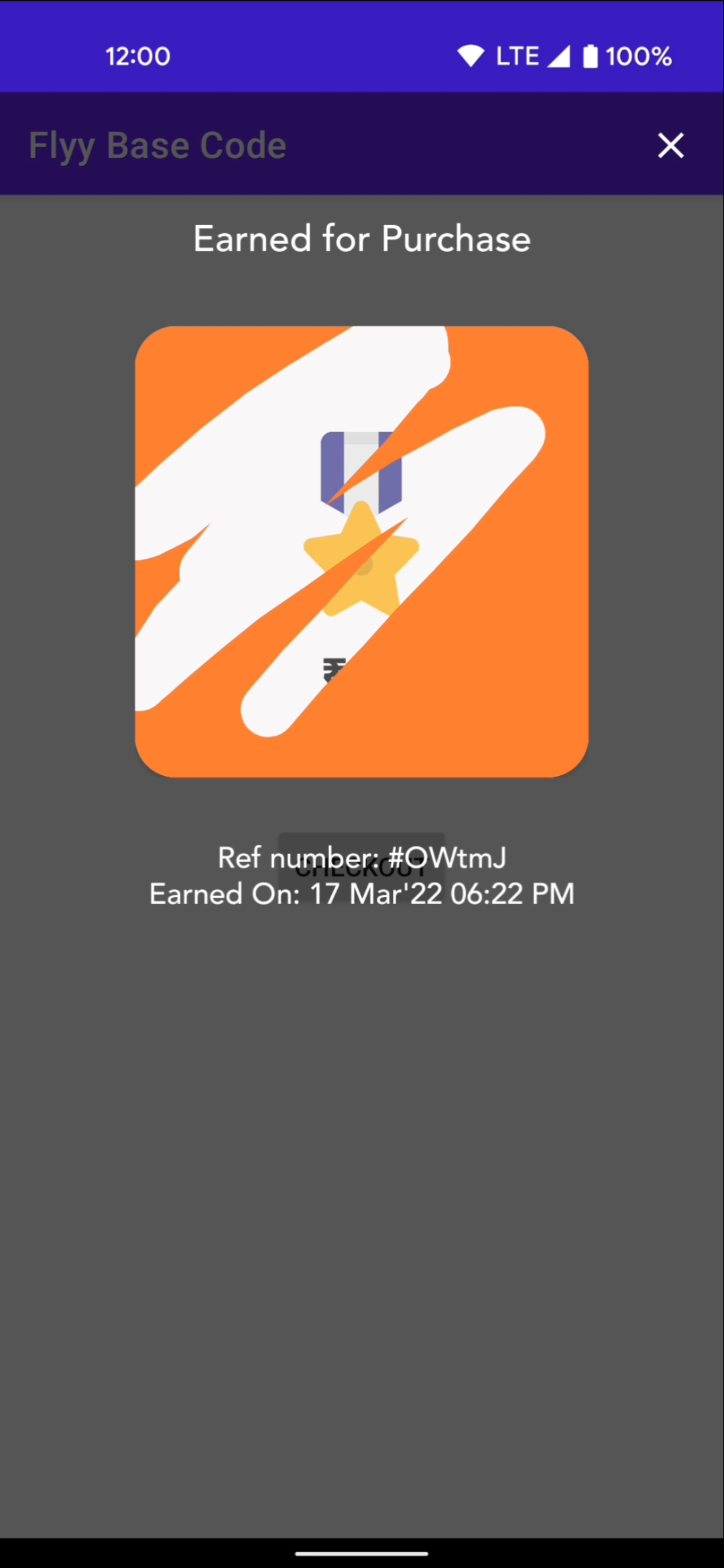
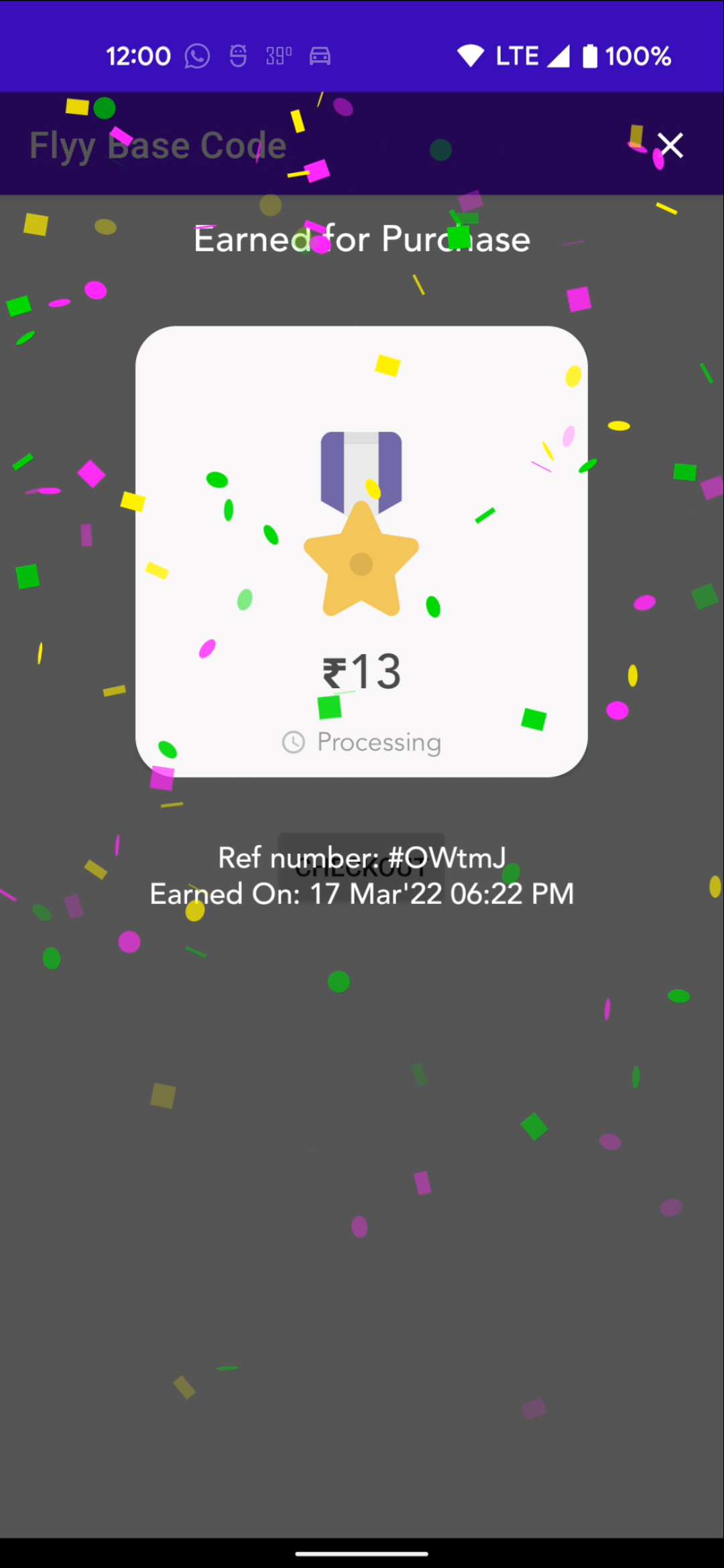
Track Campaign
View Event Status
Back in the Flyy Dashboard, go to Settings > Events. the Connection Status for the purchase event should now be Connected.
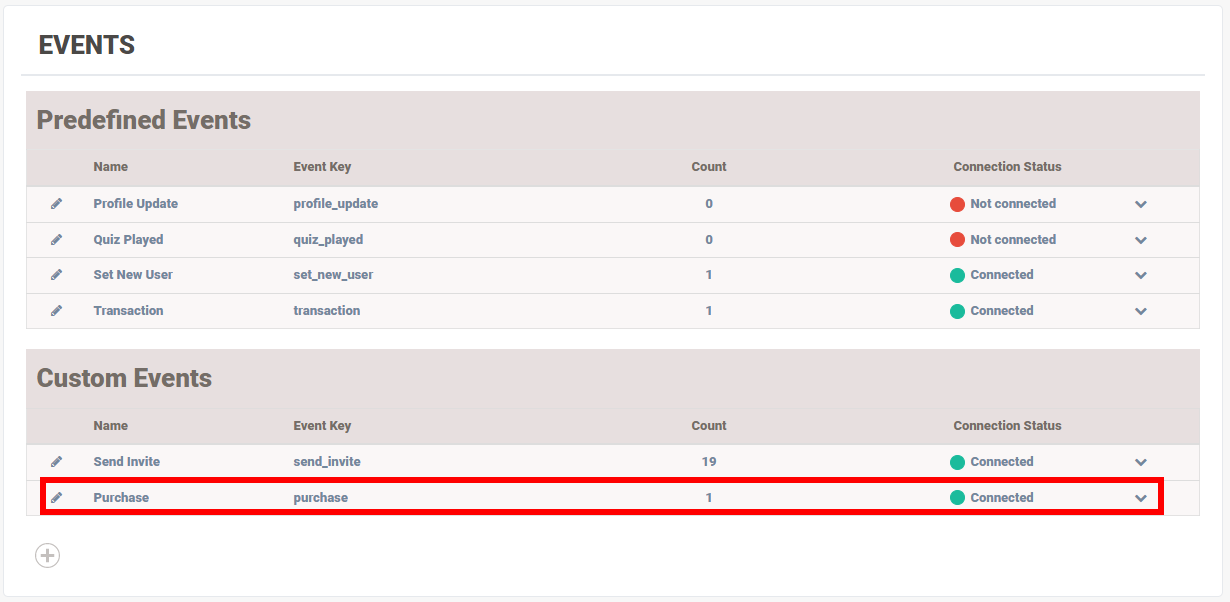
Updated almost 3 years ago