Campaign Guides
Campaign Guides for Android SDK
Invite & Earn
The following documentation contains guides for implementing and testing the Invite & Earn Campaign in an Android project.
Prerequisites
• App setup with the Flyy SDK. Setup Guide.
• An Invite & Earn Campaign running.
Overview
The referral process starts when the Referrer (User A) shares their referral link from the Offers Page on the app.
After User A has shared the link containing the Referral code, the Referee (User B) can apply the referrer's code in one of the following two ways.
- By manually typing in the referral code.
- Automatically when the app is downloaded and installed from App Store using the referral link.
Next, the user Signs Up and performs the Event to Reward, say, updating their KYC, which will complete this process and distribute the rewards.
The above makes up the complete referral process.
The referral process on User B's device is summarized in the diagram below. The developer should ensure that User B executes these steps in the exact order for a successful referral.
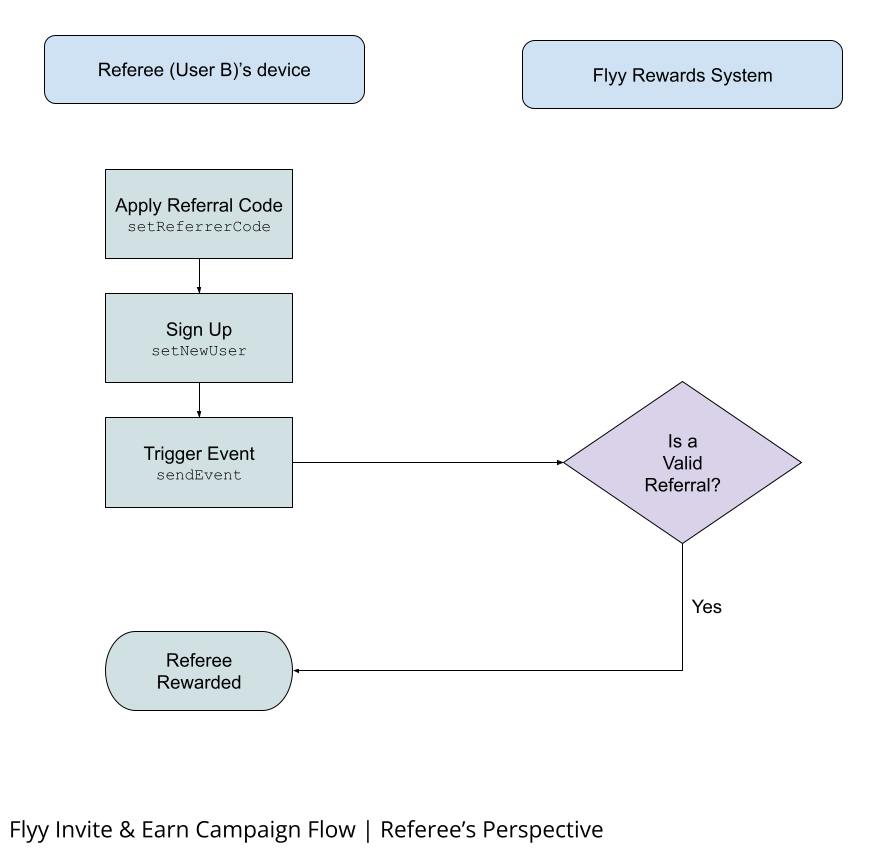
Implementation
Step 1: Initialize with FlyyReferrerDataListener
This step takes care of one of the two ways where referral code is automatically applied.
Within the init
method pass an additional argument for the FlyyReferrerDataListener
.
Use the onReferralCodeWithDataReceived
callback to perform additional UI-related actions, such as verifying the incoming referrer code. The referrer's code is automatically applied.
Flyy.init(getApplicationContext(), {partnerId}, Flyy.STAGE, new FlyyReferrerDataListener() {
@Override
public void onReferrerDataReceived(String s) {
}
@Override
public void onReferralCodeWithDataReceived(String[] strings) {
//Perform Actions when Referrer Data is received.
}
});
Flyy.init(
applicationContext,
"partnerId",
Flyy.STAGE,
object : FlyyReferrerDataListener {
override fun onReferrerDataReceived(s: String) {}
override fun onReferralCodeWithDataReceived(strings: Array<String>) {
//Perform Actions when Referrer Data is received.
}
})
Step 2: Set Referrer Code
This step takes care of the other way where the referral code is manually applied.
If the user is required to apply the referral code manually, the setReferrerCode
method should be used as follows.
However, note that the setReferrerCode
method must be called beforesetNewUser
.
Flyy.verifyReferralCode(this, refCode, new FlyyVerifyReferralCode() {
@Override
public void isReferralCodeValid(boolean isValid, String referralCode) {
if (isValid) {
Flyy.setReferrerCode(referralCode);
} else {
//Invalid referrer code.
}
}
});
Flyy.verifyReferralCode(this, refCode) { isValid, referralCode ->
if (isValid) {
Flyy.setReferrerCode(referralCode)
} else {
//Invalid referrer code.
}
}
Step 3: Sign Up
When working with the Acquisition Module campaign, it is necessary that a new user registers with the setNewUser
method instead of setUser
.
Flyy.setNewUser(userId);
Flyy.setNewUser(userId)
Step 4: Trigger Event
The final step to completing the referral process is triggering an event. The rewards will be distributed only after the campaign event is sent.
//Note: Only use the sendEvent method in Staging. For production, make
//an API call from your backend.
//See: https://docs.theflyy.com/docs/create-and-track-your-first-offer#send-event-on-checkout
Flyy.sendEvent("kyc_done", "true");
//Note: Only use the sendEvent method in Staging. For production, make
//an API call from your backend.
//See: https://docs.theflyy.com/docs/create-and-track-your-first-offer#send-event-on-checkout
Flyy.sendEvent("kyc_done", "true")
Variant Campaign Event
Follow this step only to implement the variant campaign.
From the implementation standpoint, there is just 1 difference when working with Variant Campaigns and that is the sendEvent
method expects Event Data as a JSON object.
The below code sample is about the Variant Campaign created here from the Dashboard.
//Note: Only use the sendEvent method in Staging. For production, make
//an API call from your backend.
//See: https://docs.theflyy.com/docs/create-and-track-your-first-offer#send-event-on-checkout
JSONObject object = new JSONObject();
try {
object.put("purchase", 500);
} catch (JSONException e) {
e.printStackTrace();
}
Flyy.sendEvent("invite_demo", object);
//Note: Only use the sendEvent method in Staging. For production, make
//an API call from your backend.
//See: https://docs.theflyy.com/docs/create-and-track-your-first-offer#send-event-on-checkout
val jsonObject = JSONObject()
try {
jsonObject.put("purchase", 500)
} catch (e: JSONException) {
e.printStackTrace()
}
Flyy.sendEvent("invite_demo", jsonObject)
After the 4th step, the Flyy Rewards System sends out a scratch card to the Referrer (User A) and Referee (User B).
The Flyy Rewards System performs a few checks to determine if the new users are genuine before giving out the rewards. You may run into these safeguards while testing the Invite & Earn implementation. If you do, you may find possible resolutions in the Test Invite & Earn and Troubleshooting sections.
Test Invite & Earn
Prerequisites
- Two devices (or two emulators with Google Play Services) — one for User A and one for User B.
Use the below 4 steps to test this offer in your own app or download our Invite & Earn example app.
The Flyy Rewards System requires that the mobile device used for registering a user should not have been used before for registering any other user. See the Troubleshooting section to learn how to remove users previously registered using the same device.
Testing with Your App
On User A's device
- Sign Up and check the OffersActivity screen to get the Referral Code.
The referral link will look something like this: https://stage.flyy.in/C8UFPBE
.
In this example link C8UFPBE is user A's referral code.
On User B's device
- Apply the Referral Code shared by User A.
- Perform Sign Up.
- Perform the action on User B's device that triggers the "kyc_done" event.
After the event is triggered, the Flyy Rewards System will check if this is a genuine referral and give out the reward containing a Scratch Card and notification to User A and B's devices.
Testing with the Invite & Earn Example App
Download the demo app from the Play Store. You can find its code on GitHub.
Set up FCM notifications and Play Store app redirect before starting the test.
Set up FCM Notifications
In your staging dashboard, go to Settings > SDK Keys and provide the following server key:
AAAAgM9X2Bw:APA91bGAcRPmTKjTGTESQfzUsqmCYBRnhQm4KnwuSoOpRKpn1OB5FEdqtF_CFWGMbIUyVIGecIjMwIdtx0XmYlo-dZjpLgtMLvYET9SRfr8uFzzXg441GbFyA8epdibsh3x3UKzD3glG
Redirect to the Play Store app
In your staging dashboard, go to Settings > Connect SDK and provide the following Play Store link to the app:
Now, perform the following steps.
On User A's device
- Download the App and Open it.
- On the SDK Setup screen, type in the Package Name and Partner Id as it appears in your Staging dashboard.
- Sign Up with a new User ID.
- Tap the Invite & Earn button, open the Offer, and Share the Referral Link.
On User B's device
- Tap on the Referral Link. The link should open the app listing in the Play Store. Download the app.
- Type in the Package Name and Partner Id. Click Next. A toast message indicating whether the Referral Code is valid or invalid will be displayed. If the Referral Code is valid, it is applied without needing any further steps.
- If the referral code is invalid, manually enter the referral code on the Sign-Up screen.
- Sign Up with a new User ID.
- On the Home screen, tap the UPDATE KYC button. This button is calling the
sendEvent
method with "kyc_done" as the event key in its parameter.
After tapping on the UPDATE KYC button, the Flyy Rewards System determines whether to give out the reward or not and sends a Scratch Card and notification to User A and B's devices.
Troubleshooting
If you are not receiving rewards during testing. See the common problems below.
Multiple Devices with the same GAID
Users A and B must sign up from unique devices and the same device should not exist on Flyy's dashboard before they sign up.
To verify the uniqueness of GAID, go to Settings > Playground and follow these steps:
- Perform a Search with User A's user id.
- Copy the GAID from the search result.
- Perform another search with the copied GAID.
- If more than 1 search results show up, delete all records with identical GAID.
Repeat the same steps for User B.
After you have ensured that all records with the above GAIDs have been deleted, rerun the test.
Notification Issue
Go to Reports > Referrals and check if the new referral record with User A and B record is created. If the new record exists, but the devices do not receive a notification, test your app's FCM configuration.
Also, ensure if the correct Server Key has been provided at Settings > SDK Keys.
To test notifications from the dashboard.
- Go to Settings > Playground from the dashboard.
- Search by User Id.
- Click "Test FCM". Note that at least 1 Campaign must be active.
Referral Links do not Redirect to Play Store Listing
Ensure that redirect is configured.
Updated almost 3 years ago