SDK Documentation
Overview of all the SDK features available
Primary Screens
Offers Screen
To open the offers screen for the user.
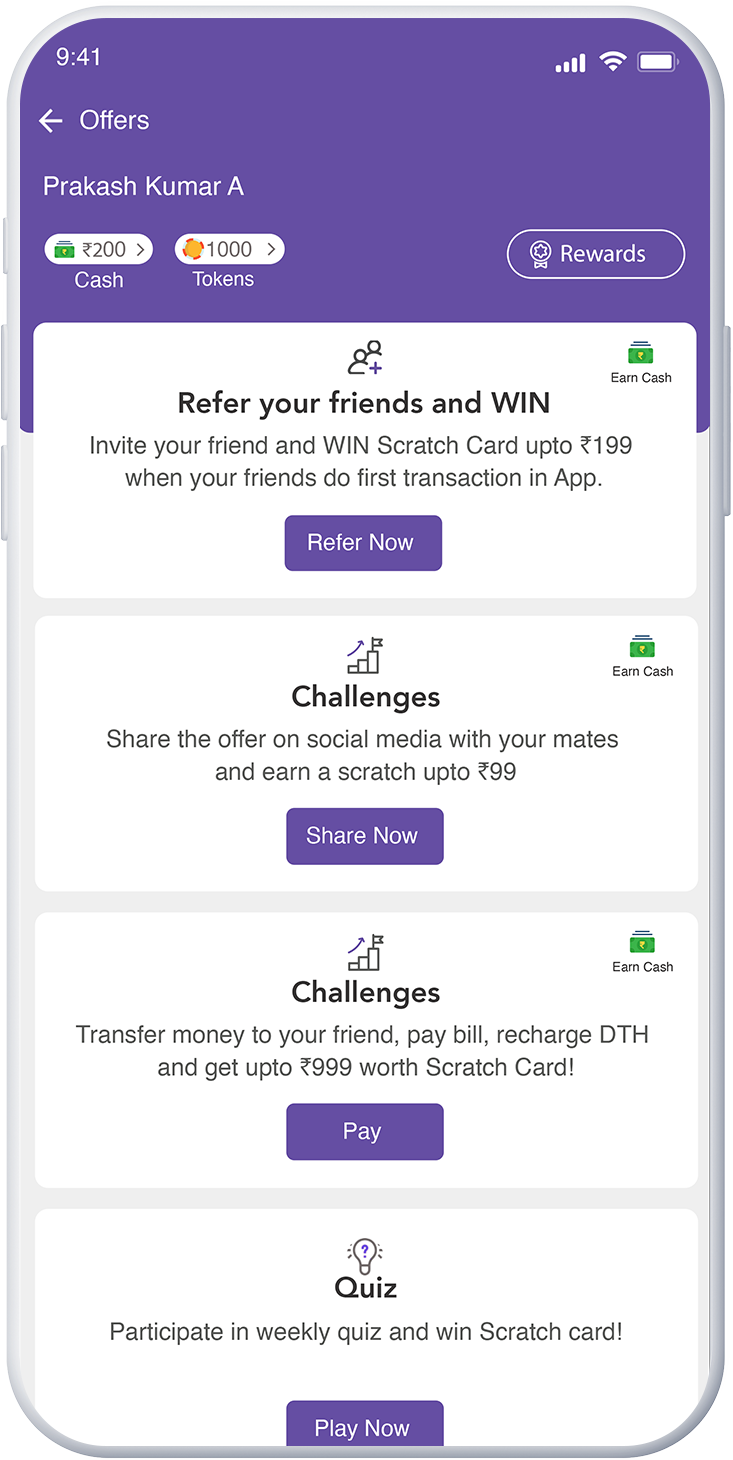
FlyyFlutterPlugin.openFlyyOffersPage();
Rewards Screen
To open rewards screen for user.
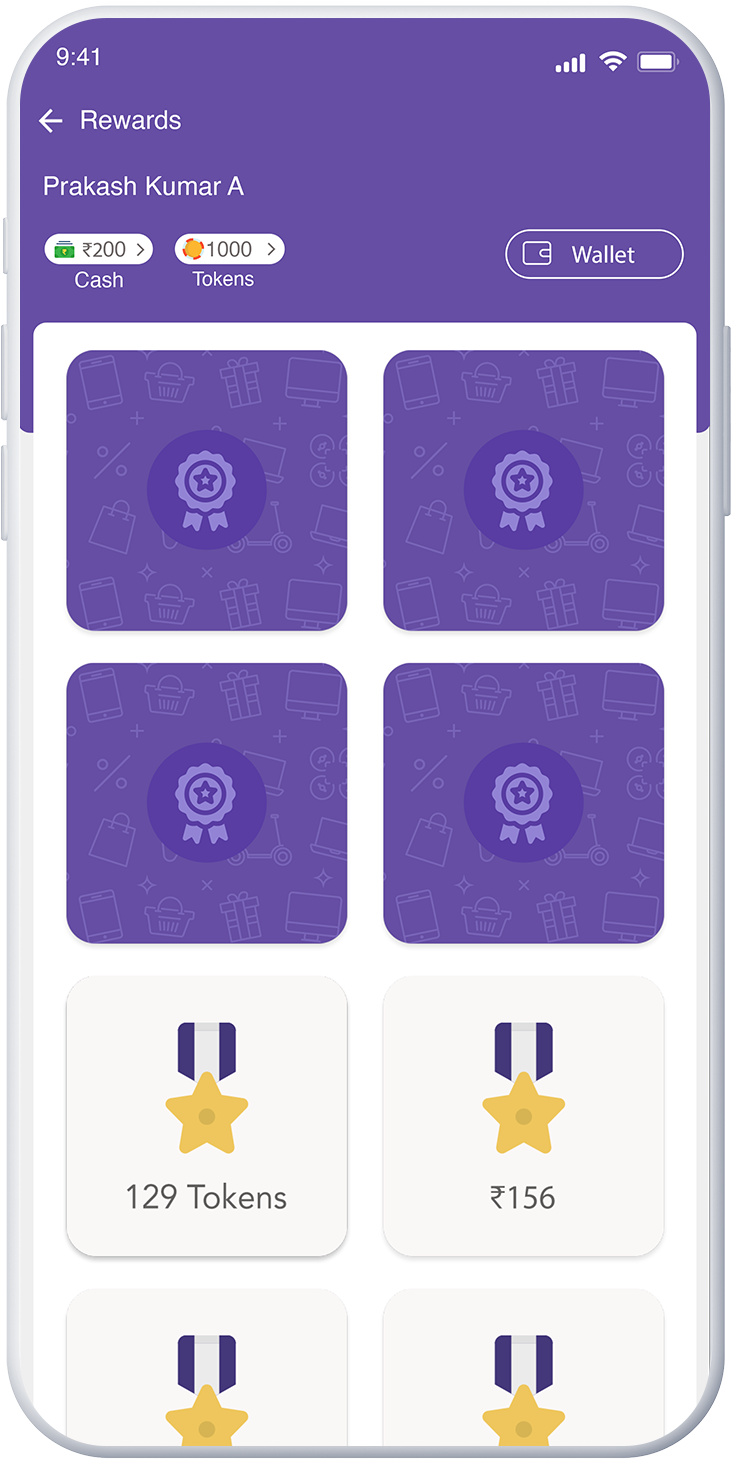
FlyyFlutterPlugin.openFlyyRewardsPage();
Wallet Screen
To open wallet screen for user
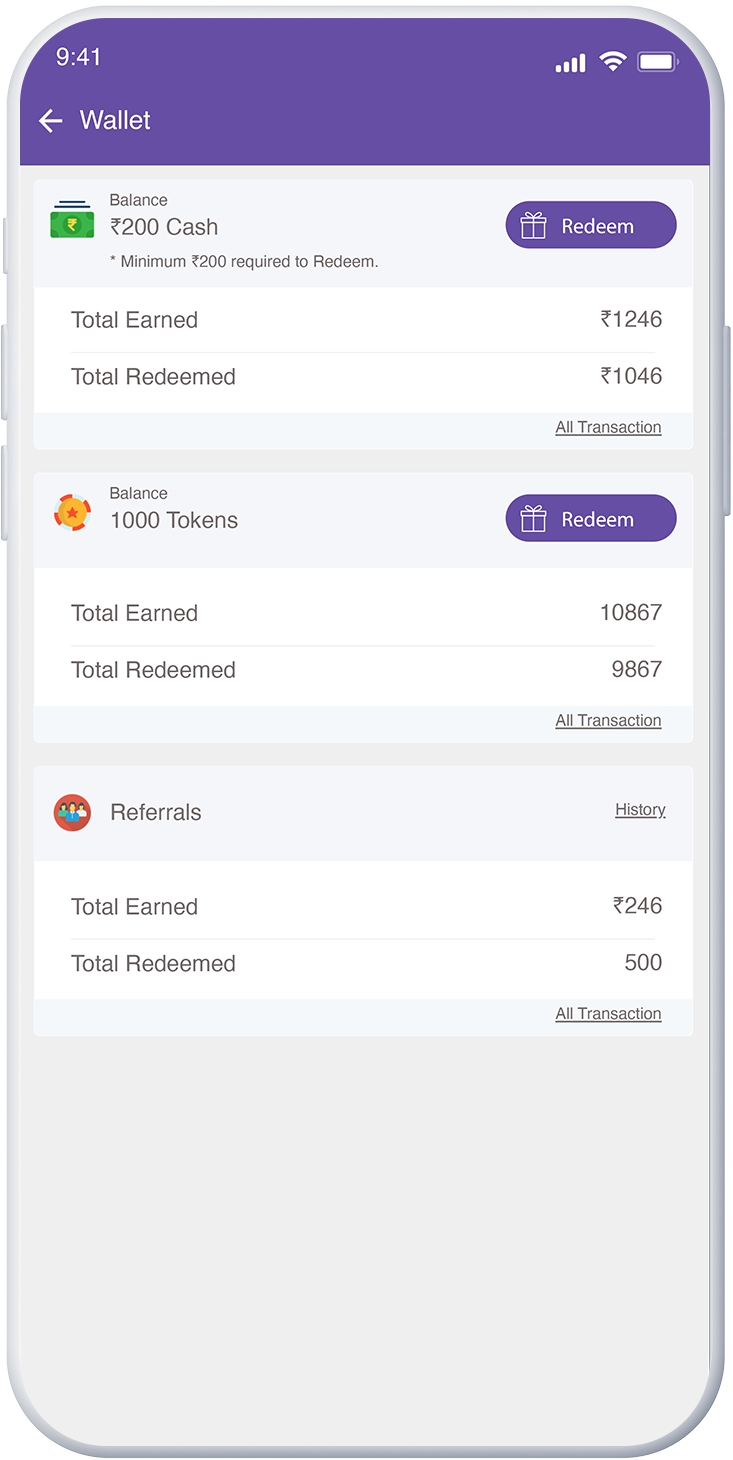
FlyyFlutterPlugin.openFlyyWalletPage();
Additional Screens
Gift Cards Screen
To open the gift cards screen for the user
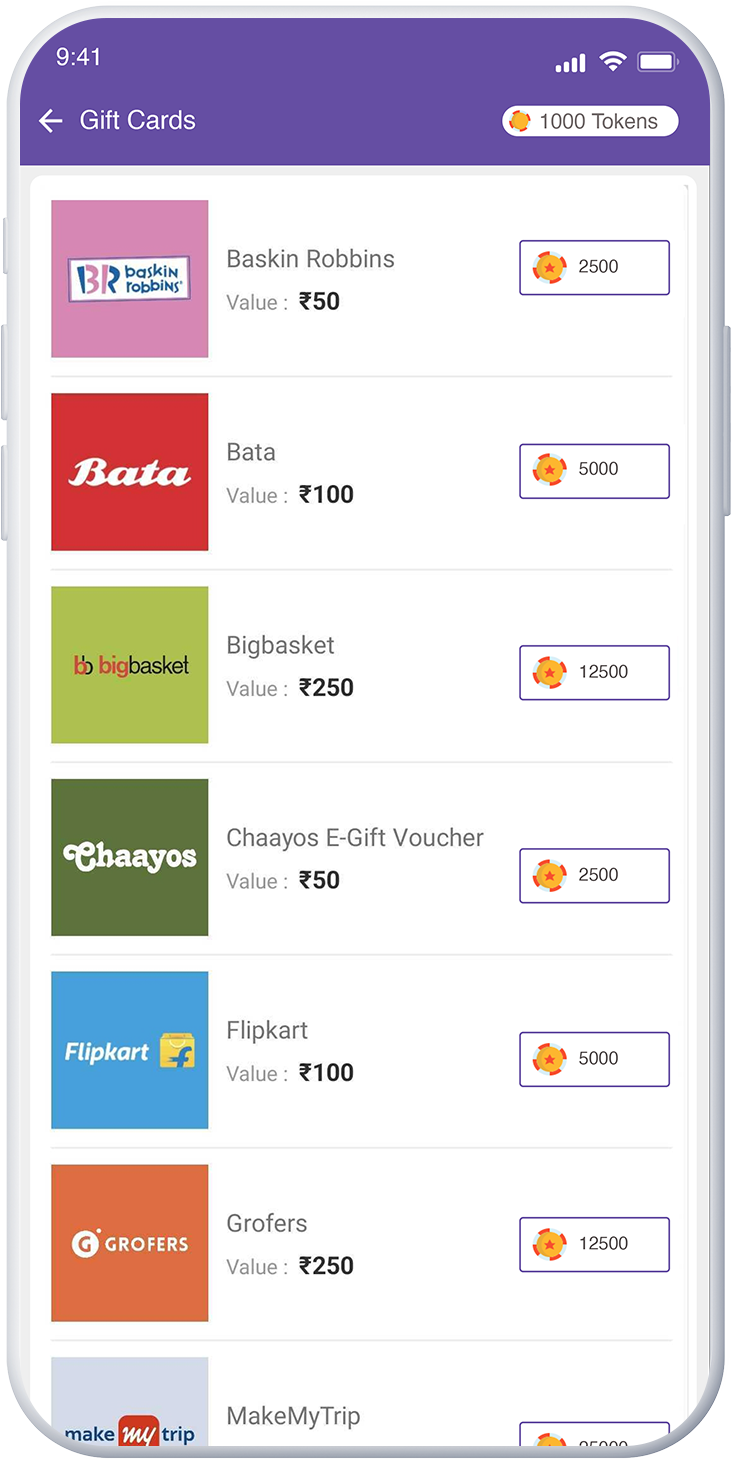
FlyyFlutterPlugin.openFlyyGiftCardsPage();
Referral History Screen
To open the referral history screen for the user
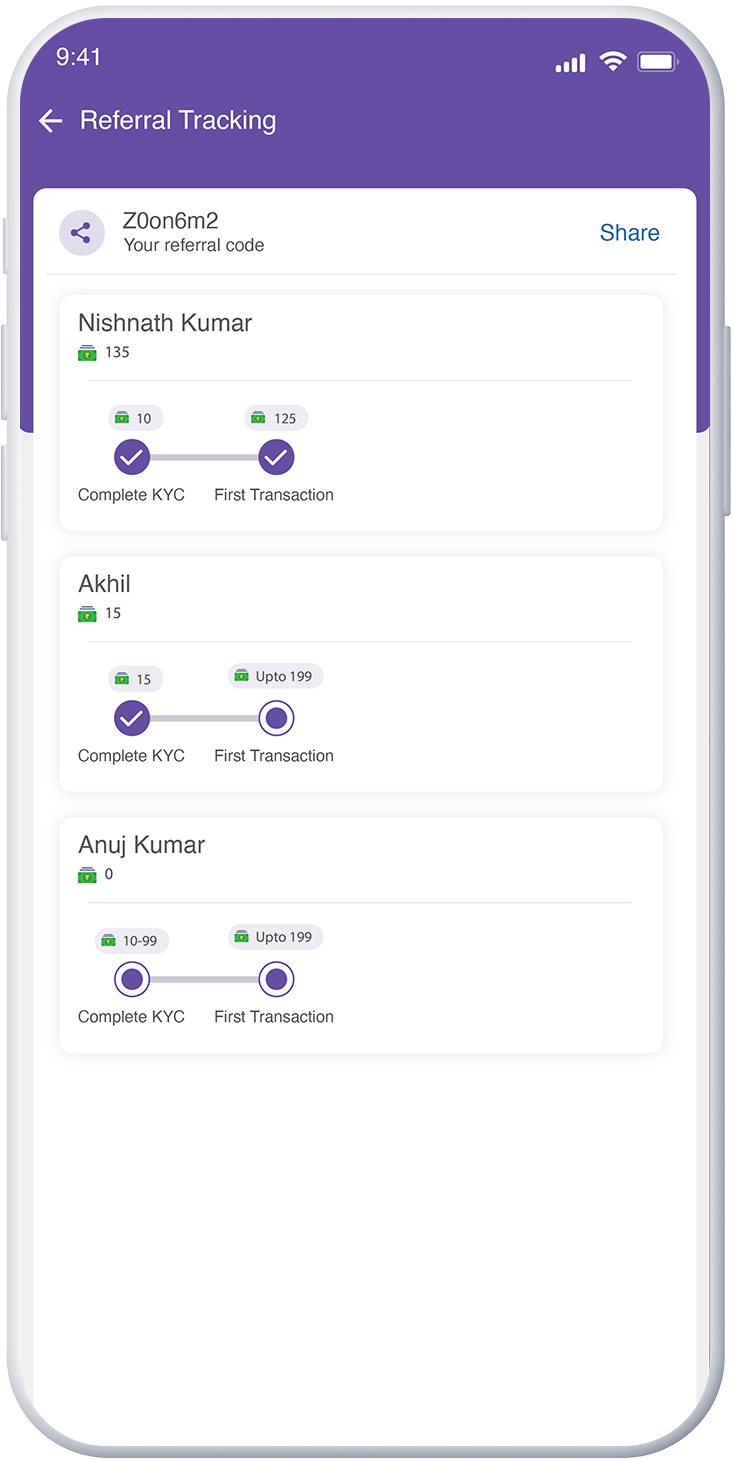
FlyyFlutterPlugin.openFlyyReferralsPage();
Quiz Screen
To open quiz screen for the user
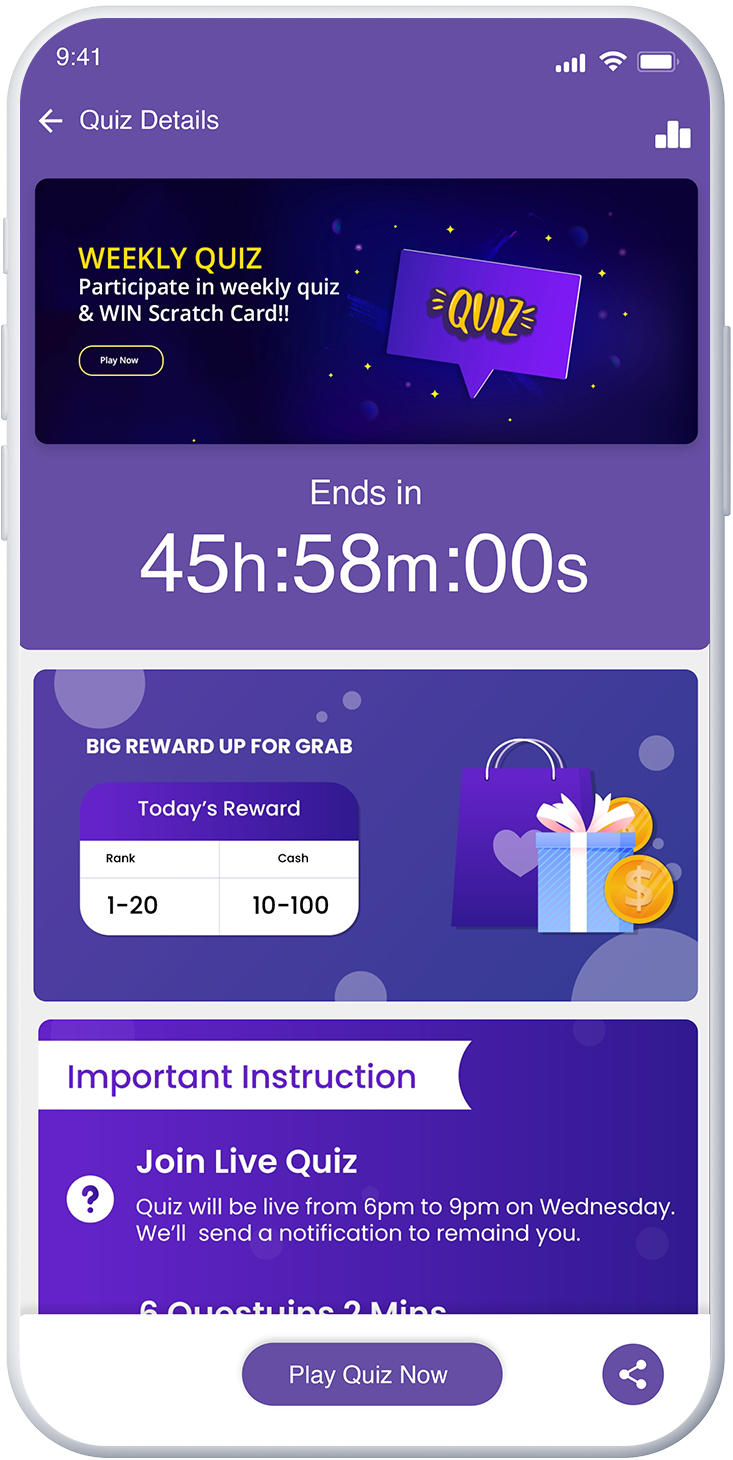
FlyyFlutterPlugin.openFlyyQuizPage(0);
//live quiz_id or 0
Quiz History Screen
To open the quiz history screen for the user
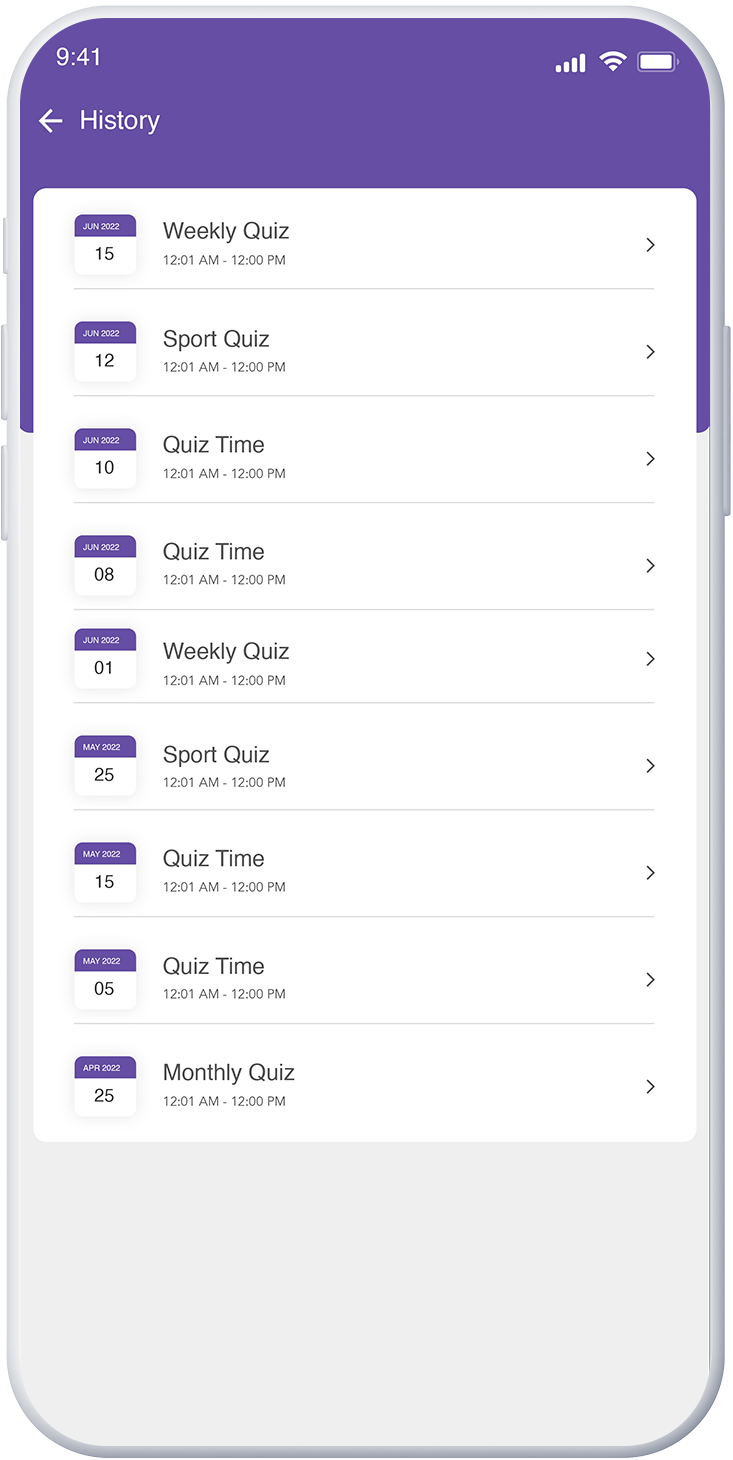
FlyyFlutterPlugin.openFlyyQuizHistoryPage();
Quiz List Screen
To open the quiz list screen for the user.
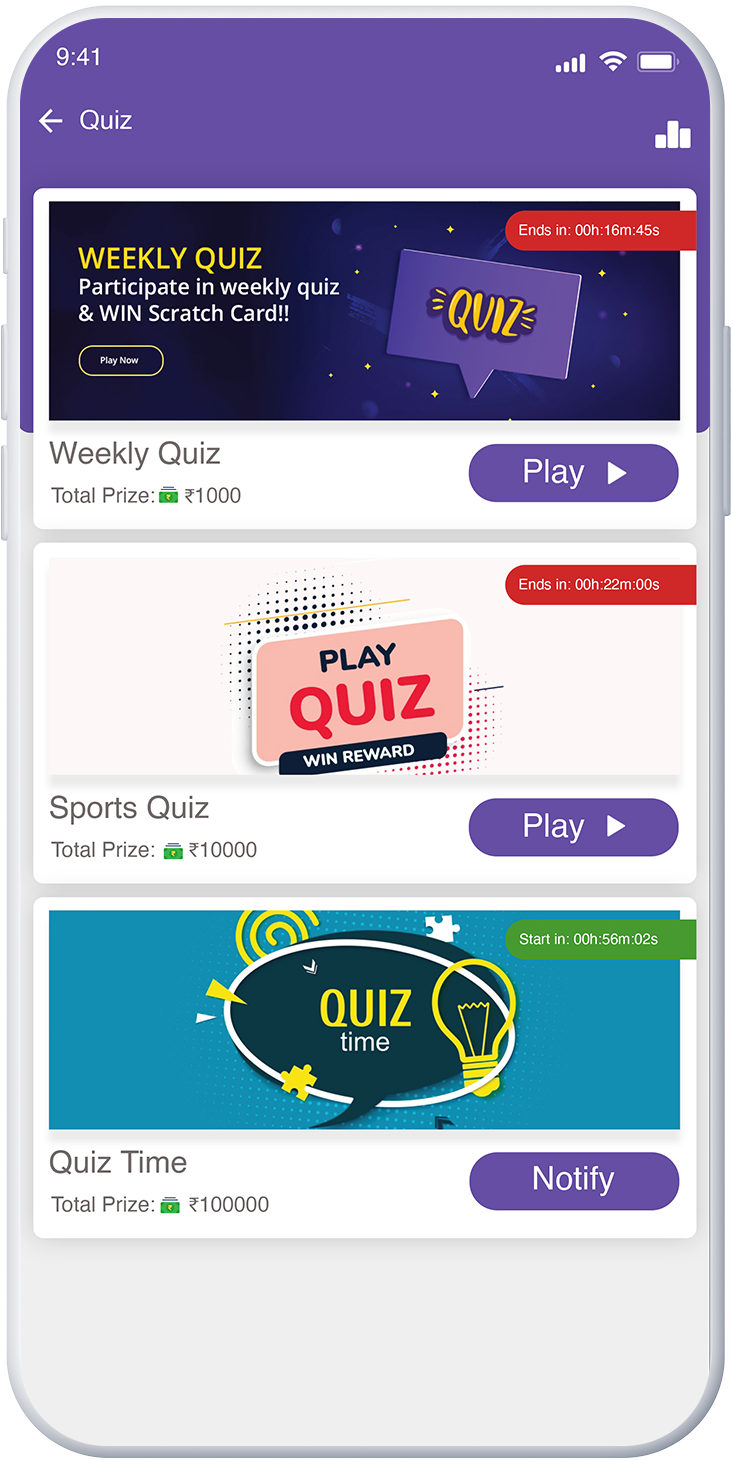
FlyyFlutterPlugin.openFlyyQuizListPage();
Stamps Screen
To open the stamps screen for the user.
FlyyFlutterPlugin.openFlyyStampsPage();
Invite Detail Screen
To open the invite detail screen for the user.
FlyyFlutterPlugin.openFlyyInviteDetailsPage(offer_id);
//offer_id can be null
Invite And Earn Screen
To open the invite and earn screen for the user.
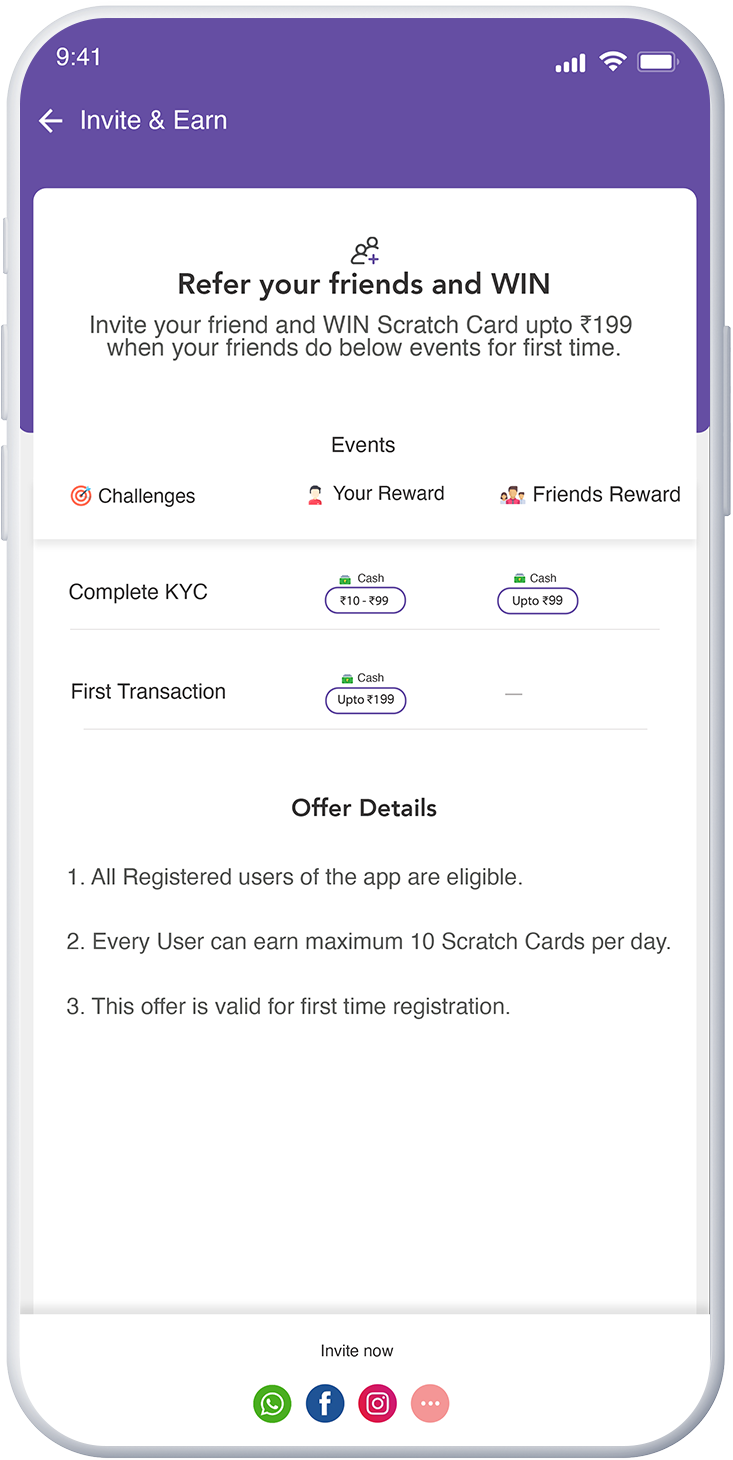
FlyyFlutterPlugin.openFlyyInviteAndEarnPage(offer_id);
//offer_id can be null
Custom Invite and Earn Screen
To open a custom invite and earn screen for the user.
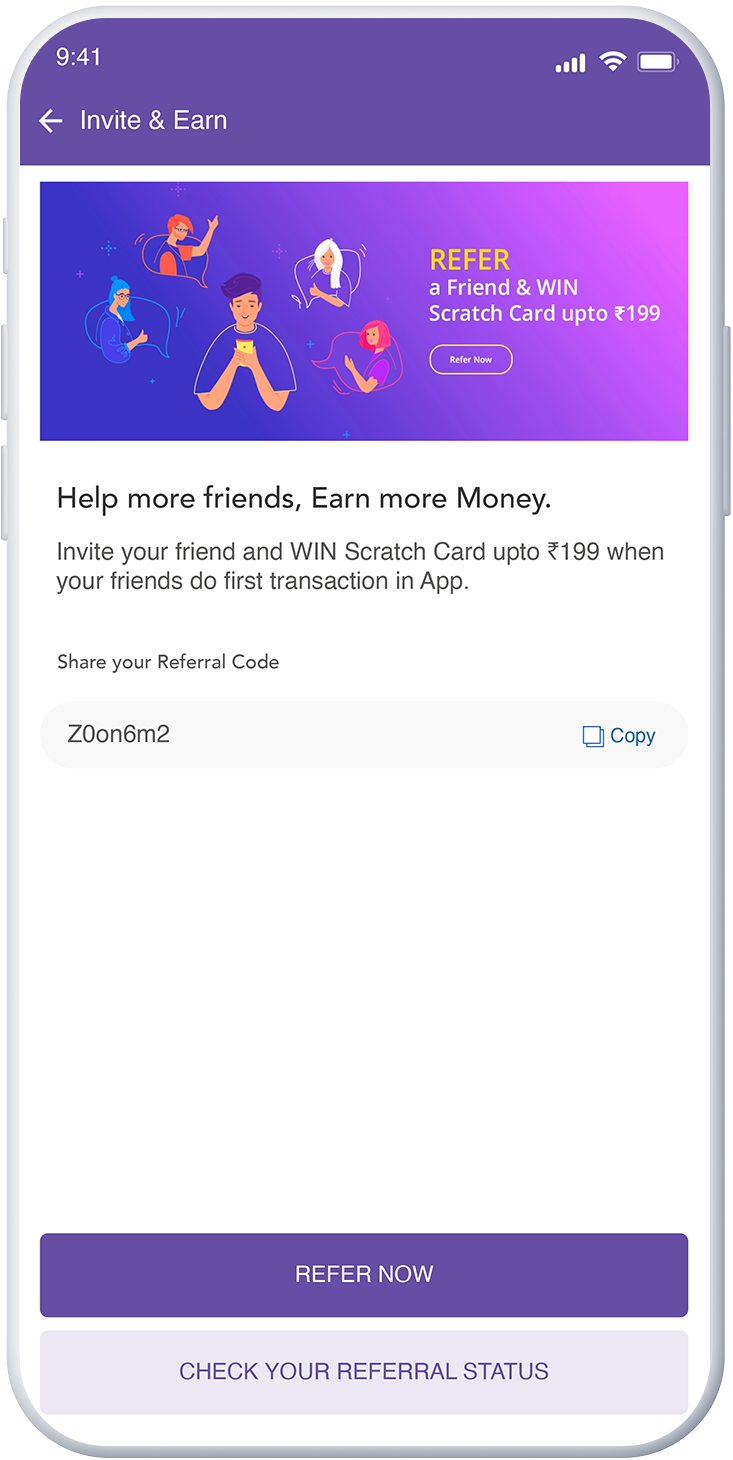
FlyyFlutterPlugin.openFlyyCustomInviteAndEarnPage(offerId, "#ffffff");
//offer_id can be null
//tool bar items default color will be black if color is not passed
Challenge Details Screen
To open the challenge details screen for the user.
FlyyFlutterPlugin.openFlyyChallengeDetailsPage(offer_id);
//offer_id can be null
Bonanza Screen
To open a bonanza details screen for user.
FlyyFlutterPlugin.openFlyyBonanzaPage();
Additional Methods
Set Theme Colors
The Flyy SDK inherits theme colors which are provided to the setThemeColor method. The setThemeColor method must be called immediately after the init method is called.
//Parameters to be passed are colorPrimary and colorPrimaryDark
FlyyFlutterPlugin.setThemeColor("#FF5733", "#842C19");
Set Name
This will be shown in the screens wherever username is displayed.
FlyyFlutterPlugin.setFlyyUserName("<user_name>");
SDK Initialization with referral callback
Initialize SDK in your application or main entry point of the app with referral callback as shown below.
//For Production
String referrerData = await FlyyFlutterPlugin.initFlyySDKWithReferralCallback("<partner_id>", FlyyFlutterPlugin.PRODUCTION);
//For Staging
String referrerData = await FlyyFlutterPlugin.initFlyySDKWithReferralCallback("<partner_id>", FlyyFlutterPlugin.STAGE);
Set User Token
To increase security for your users you can set unique user token in the following manner instead of setUser() method
//without callback
FlyyFlutterPlugin.setFlyyUserToken("BSt4Y3A2"); //pass your user token
Set User Token along with Segment ID
To increase security for your users you can set unique user token along with segment id in the following manner instead of setUser() method
//without callback
FlyyFlutterPlugin.setFlyyUserTokenWithSegment("BSt4Y3A2", "all_users");
Set New User Token
To increase security for your users you can set unique new user token for new registered users in the following manner instead of setNewUser() method
FlyyFlutterPlugin.setFlyyNewUserToken("QWt4Y3A2"); //pass your new user token
Set New User Token along with Segment ID
To increase security for your users you can set unique new user token for new registered users along with segment id in the following manner instead of setNewUser() method
//without callback
FlyyFlutterPlugin.setFlyyNewUserTokenWithSegment("QWt4Y3A2", "all_users");
Set Bank Details
You can use the following method to set user redemption details, which takes following params,
- Account number - bank account number
- IFSC code
- Name
FlyyFlutterPlugin.setBankDetails("0000xxxxxxxxxx16", "BSxxxxxxx0", "user name");
Set UPI Details
You can use the following method to set user redemption details, which takes following params,
- UPI ID
FlyyFlutterPlugin.setUpiDetails("user@upiId");
Send Events
Sending User Event with API
The
sendEvent
method should only be used for testing on the Staging environment.
For production, use the Sending User Event API call from your backend instead. AllsendEvent
events generating from the front-end are ignored by the Flyy's reward system.
Values can either be sent in the form of key and value or as a json object.
FlyyFlutterPlugin.sendEvent("key", "value");
//OR
FlyyFlutterPlugin.sendJSONEvent("registration", Map<String, String>);
Set Referral Code
To set referral code, please call the following function
FlyyFlutterPlugin.setFlyyReferralCode("sdfhyr6");
Get Referral Code
To get the referral code, please call the following function
String referralCode = await FlyyFlutterPlugin.getFlyyReferralCode();
Verify Referral Code
To verify if referral code is valid or not, please call the following function
await FlyyFlutterPlugin.verifyReferralCode("asdkjjkj23");
//To get result
print(mapResult["is_valid"]);
print(mapResult["referral_code"]);
Show the Notification Popup
Use the below code to show a default Flyy notification popup. You can keep the notificaiton_id and campaign_id 0/-1 if you don't have one.
FlyyFlutterPlugin.showNotififactionPopup(int notificationId, String title, String message, String bigImage, String deeplink, int campaignId);
Show Reward Won Popup
Use the below code to show the reward won popup.
FlyyFlutterPlugin.showRewardWonPopup(String title, String message, String deeplink, String buttonText, bool showConfetti);
Show Reward Won Scratch Card Popup
Use the below code to show the reward won scratch card popup.
FlyyFlutterPlugin.showRewardWonScratchPopup(String title, String message, bool showConfetti, String refNum);
Get share data
Use the below code to get share data. In the callback you will receive following params
- referral_link - Referral link to share as string
- referral_message - Referral message to share as string
- Share_message - Share message as string
try {
Map<String, dynamic> mapShareData =
await FlyyFlutterPlugin.getShareData();
//To get result
print(mapShareData["referral_link"]);
print(mapShareData["referral_message"]);
print(mapShareData["share_message"]);
} on PlatformException catch (e) {
print(e.message);
}
Get Referral Count
Use the below code to get the referral count. In the callback, you will receive the referral count as an integer.
try {
int referralCount = await FlyyFlutterPlugin.getReferralCount();
//To get result
print(referralCount);
} on PlatformException catch (e) {
print(e.message);
}
Get Scratch Card Count
Use the below code to get the scratch card count.
try {
Map<String, dynamic> mapShareData =
await FlyyFlutterPlugin.getScratchCardCount();
//To get result
print(mapShareData["total_sc_count"]);
print(mapShareData["scratched_sc_count"]);
print(mapShareData["unscratched_sc_count"]);
print(mapShareData["locked_sc_count"]);
} on PlatformException catch (e) {
print(e.message);
}
Get Previous Leaderboard Winner
Use the below code to get previous leaderboard winners.
try {
Map<String, dynamic> mapShareData =
await FlyyFlutterPlugin.getPreviousLeaderboardWinners("<tag>");
//To get result
print(mapShareData["participants_count"]);
print(mapShareData["winners"]);
print(mapShareData["previous_winners"]);
} on PlatformException catch (e) {
print(e.message);
}
Get Leaderboard Participants
Use the below code to get leaderboard participants.
try {
Map<String, dynamic> mapShareData =
await FlyyFlutterPlugin.getLeaderboardParticipants("<tag>");
//To get result
print(mapShareData["participants_count"]);
print(mapShareData["winners"]);
print(mapShareData["previous_winners"]);
} on PlatformException catch (e) {
print(e.message);
}
Get Wallet Balance
Use the below code to get wallet balance.
try {
Map<String, dynamic> mapShareData =
await FlyyFlutterPlugin.getWalletBalance("cash");
//To get result
print(mapShareData["balance"]);
print(mapShareData["total_credit"]);
print(mapShareData["total_debit"]);
} on PlatformException catch (e) {
print(e.message);
}
Get Referrer Details
Use the below code to get referrer details.
try {
Map<String, dynamic> mapShareData1 =
await FlyyFlutterPlugin.getReferrerDetails();
//To get result
print(mapShareData1["name"]);
print(mapShareData1["ext_uid"]);
print(mapShareData1["extra_data"]);
} on PlatformException catch (e) {
print(e.message);
}
Get Offers Count
Use the below code to get offers count.
try {
Map<String, dynamic> mapShareData2 =
await FlyyFlutterPlugin.getOffersCount();
//To get result
print(mapShareData2["live_offers_count"]);
print(mapShareData2["participated_offers_count"]);
} on PlatformException catch (e) {
print(e.message);
}
Advanced Implementation
Add User to a Segment
To add a user to a specific segment. If you are adding a user to an existing segment, you can pass null in segment_title. If the user segment is not present it will create a new one
FlyyFlutterPlugin.addUserToSegment(String segmentTitle, String segmentKey);
Get Screen Visits data
Use the below code to get the following data:-
[“home_checkin_clicked”, “offer_card_clicked”, “game_card_clicked”, “quiz_card_clicked”, “stamp_campaign_card_clicked”, “leaderboard_card_clicked”, “checkin_card_clicked”, “challenges_card_clicked”, “raffle_card_clicked”, “transaction_screen_visited”, “detail_checkin_clicked”, “referrals_screen_visited”, “rewards_screen_visited”, “wallet_screen_visited”]
Map<String, dynamic> events = await FlyyFlutterPlugin.trackUIEvents();
Updated over 2 years ago