SDK Documentation
Primary Screens
Offers Screen
To open offers a screen for the user.
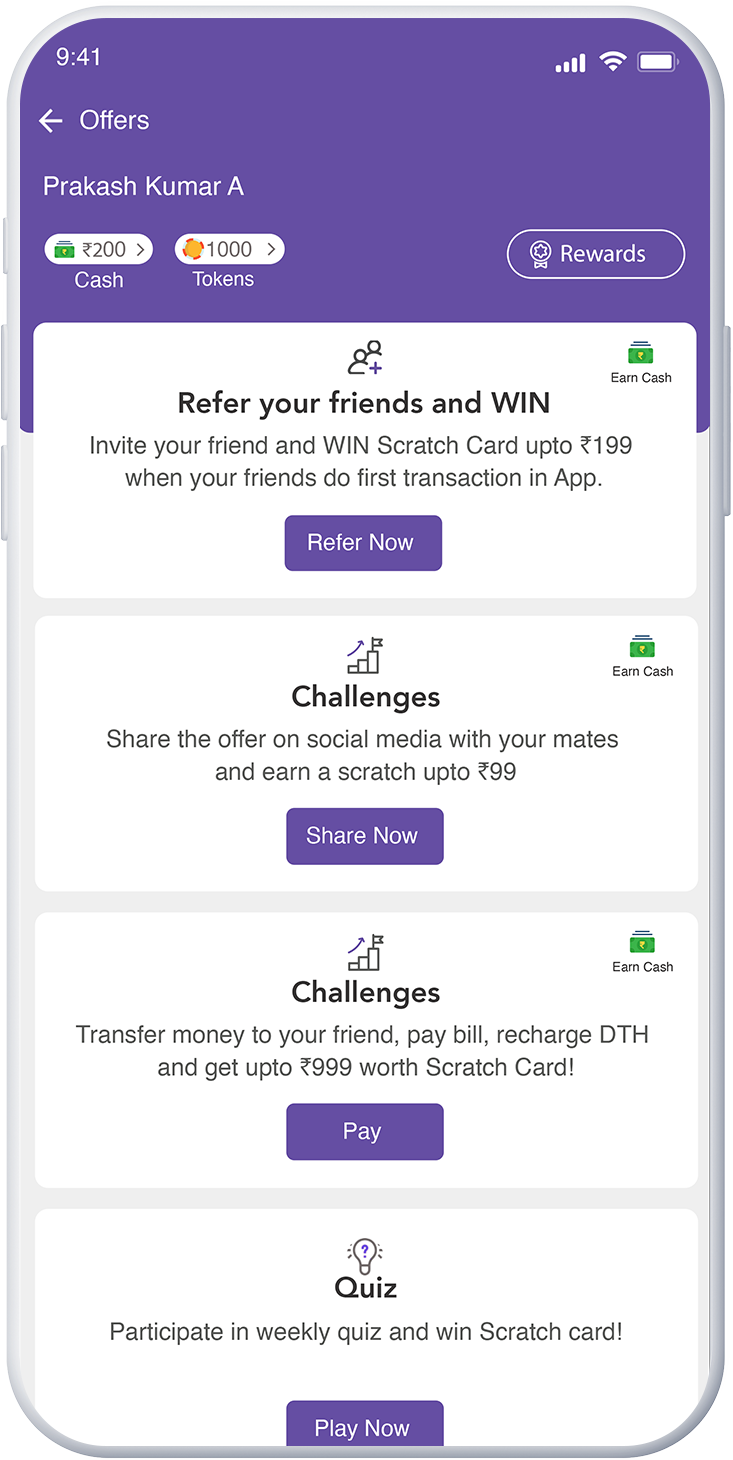
Flyy.sharedInstance.openOffersPage()
[flyy openOffersPage];
Rewards Screen
To open rewards screen for user
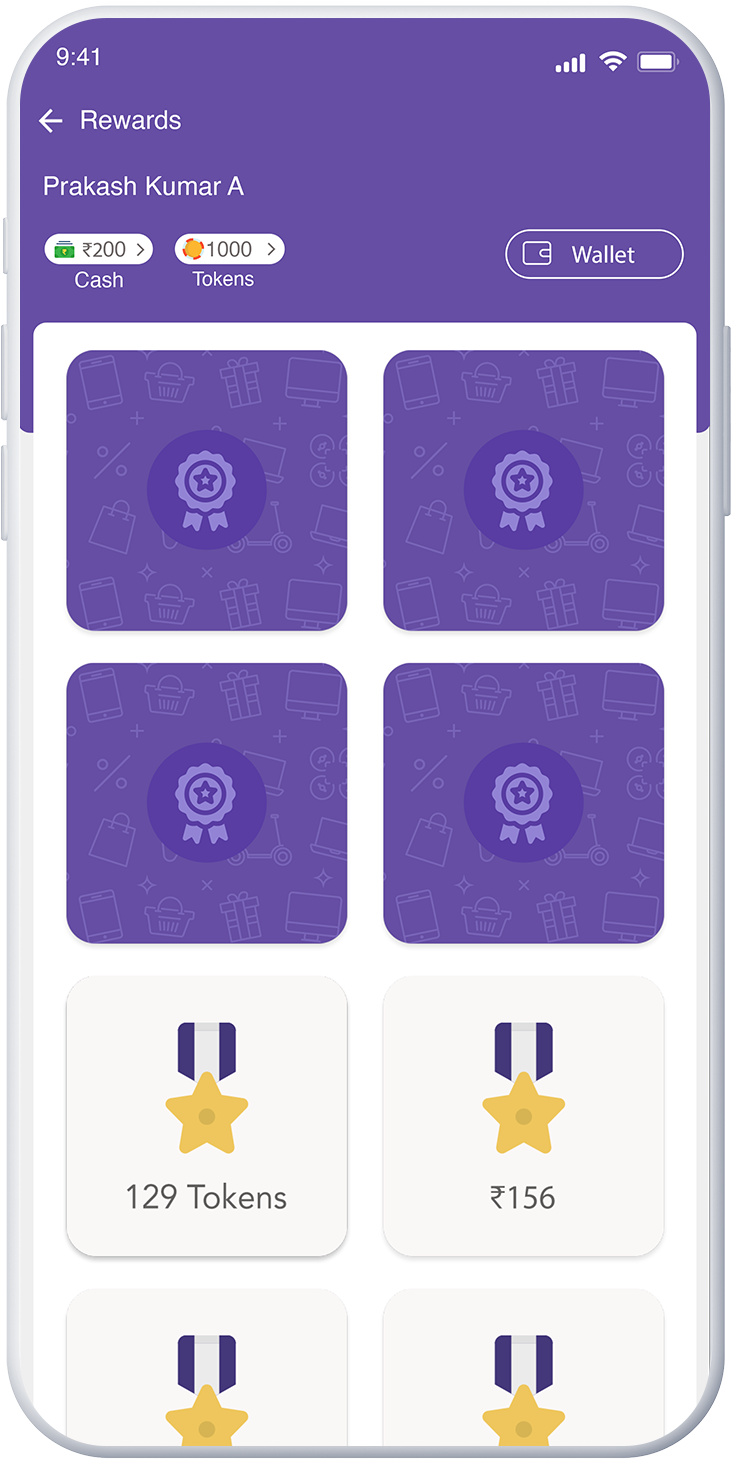
Flyy.sharedInstance.openRewardsPage()
[flyy openRewardsPage];
Wallet Screen
To open wallet screen for user
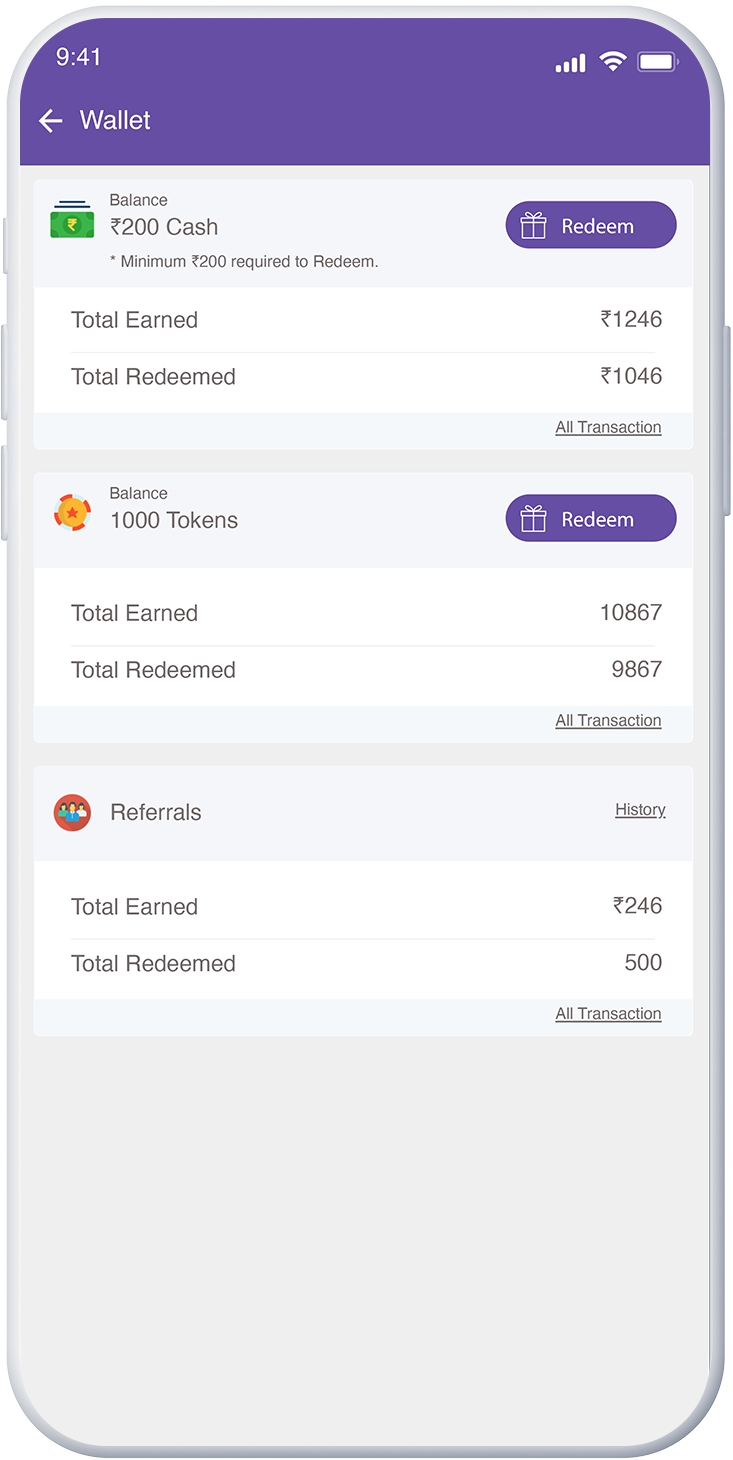
Flyy.sharedInstance.openWalletPage()
[flyy openWalletPage];
Additional Screens
Gift Cards Screen
To open gift cards screen for user.
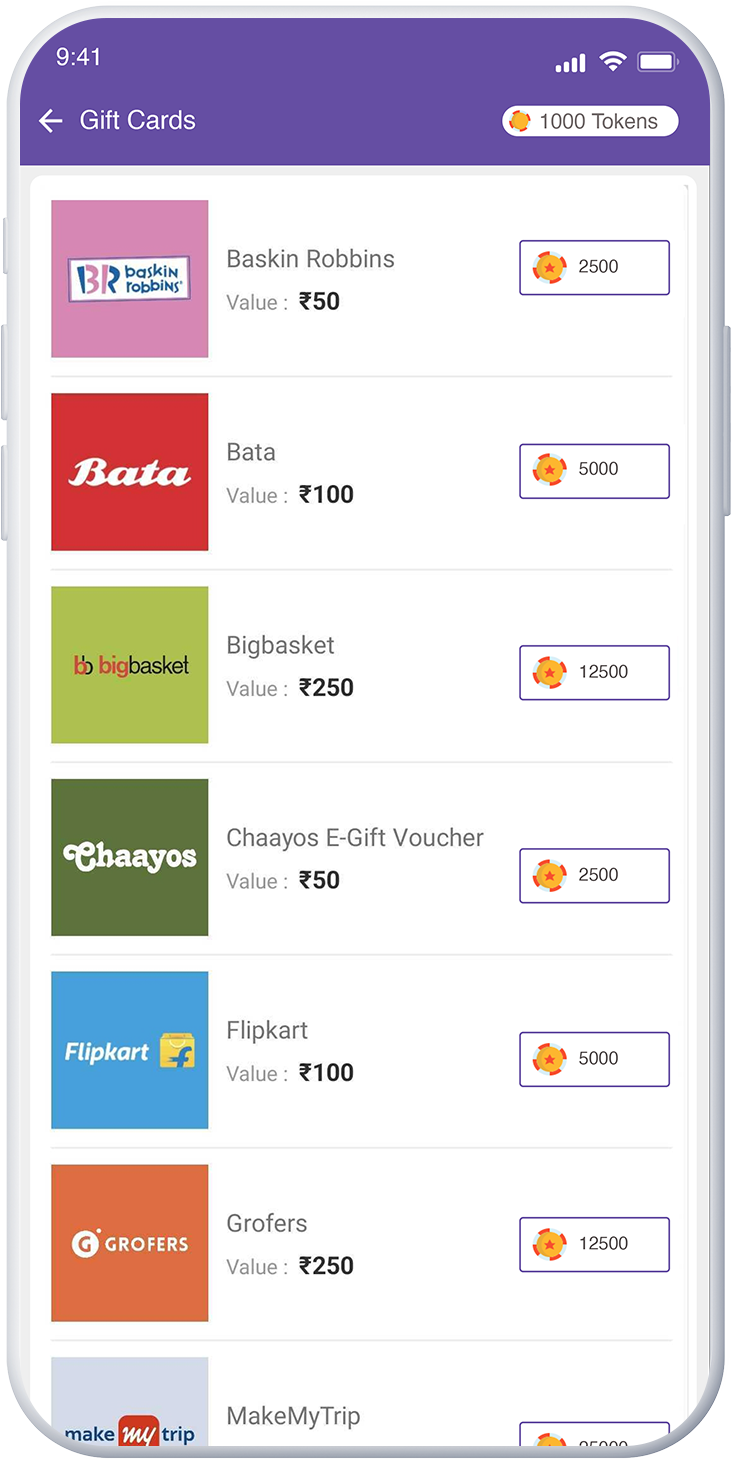
Flyy.sharedInstance.openGiftCardPage()
[flyy openGiftCardPage];
Referral History Screen
To open referral history screen for user.
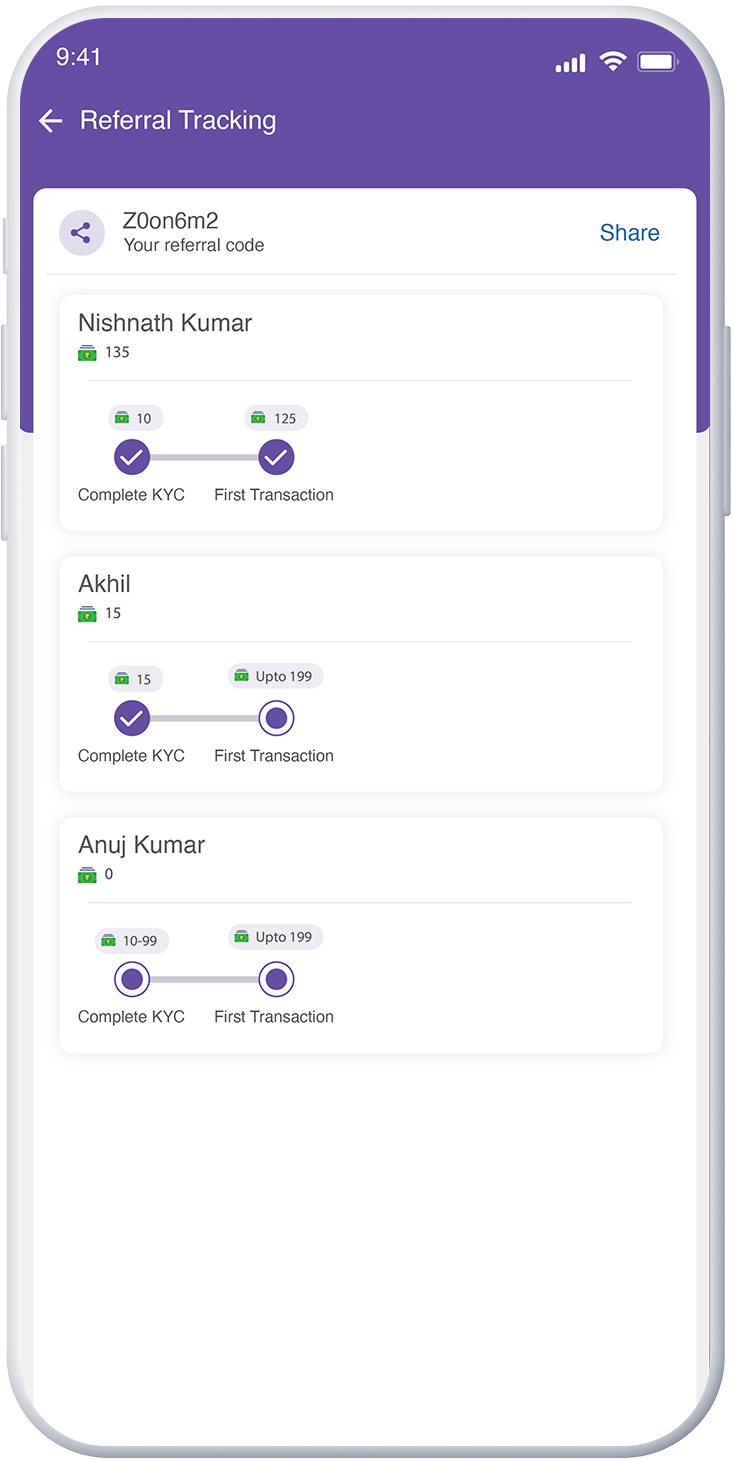
Flyy.sharedInstance.openReferralHistoryPage()
[flyy openReferralHistoryPage];
Quiz Screen
To open quiz screen for user.
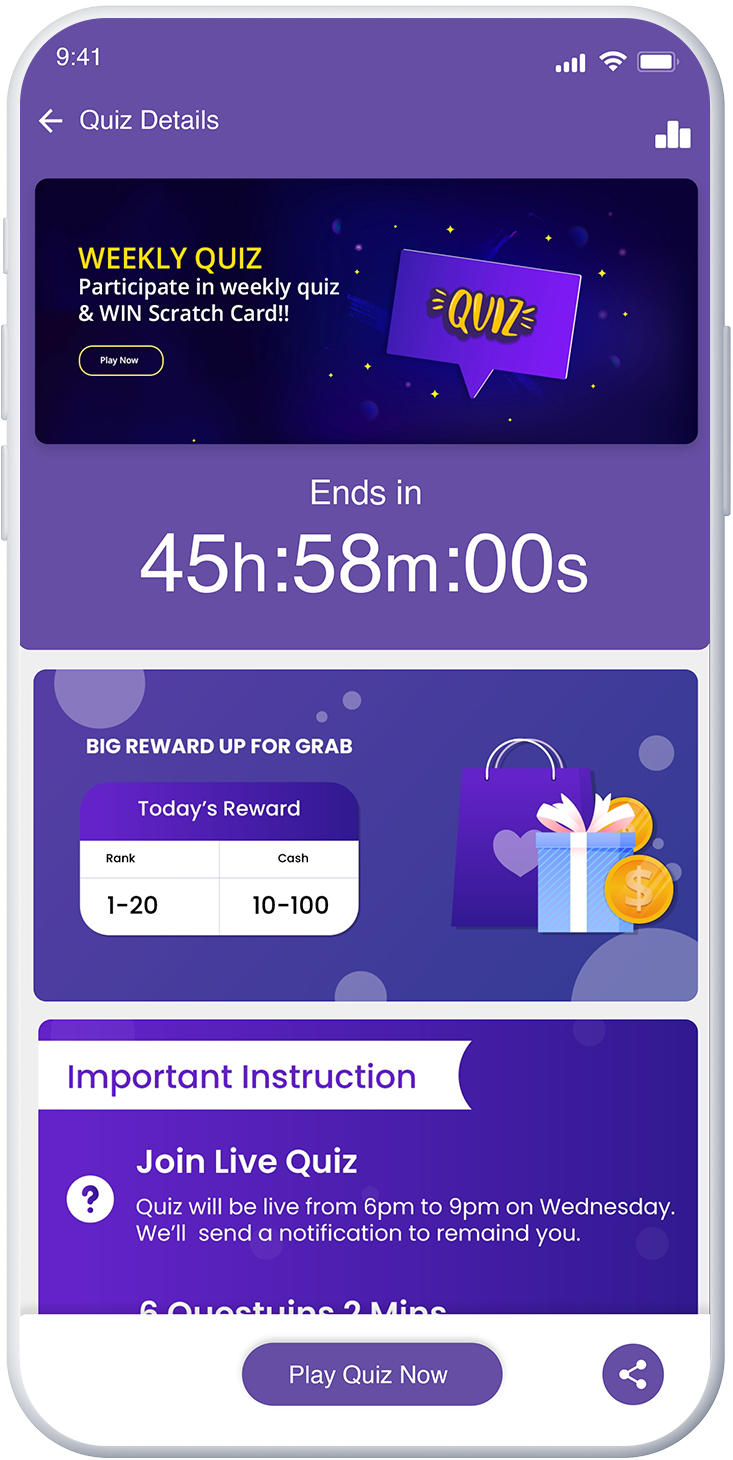
//Live quiz_id or 0
Flyy.sharedInstance.openQuizListDetailPage(quizId: 0)
//Live quiz_id
[flyy openQuizListDetailPageWithQuizId:0];
Quiz History Screen
To open quiz history screen for user.
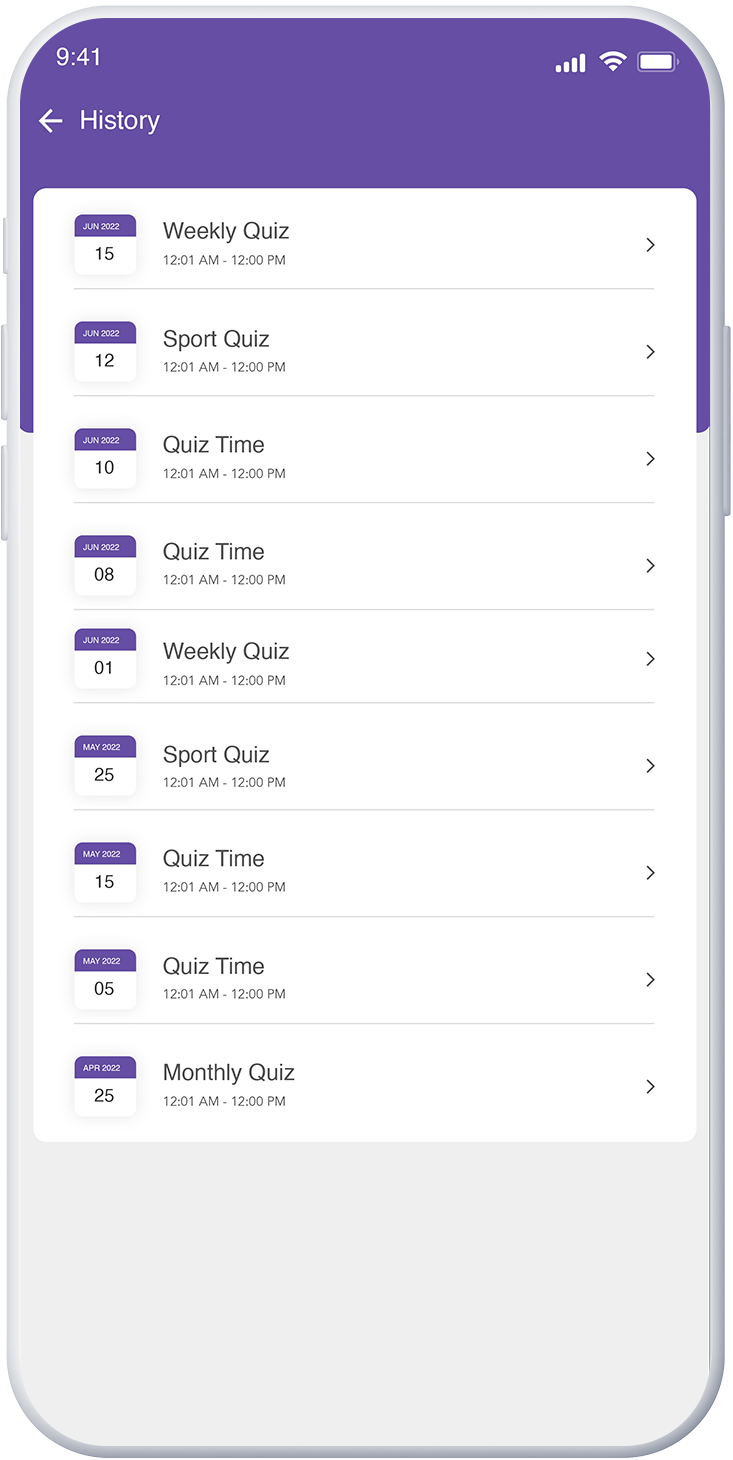
Flyy.sharedInstance.openQuizHistoryPage()
[flyy openQuizHistoryPage];
Quiz List Screen
To open quiz list screen for user.
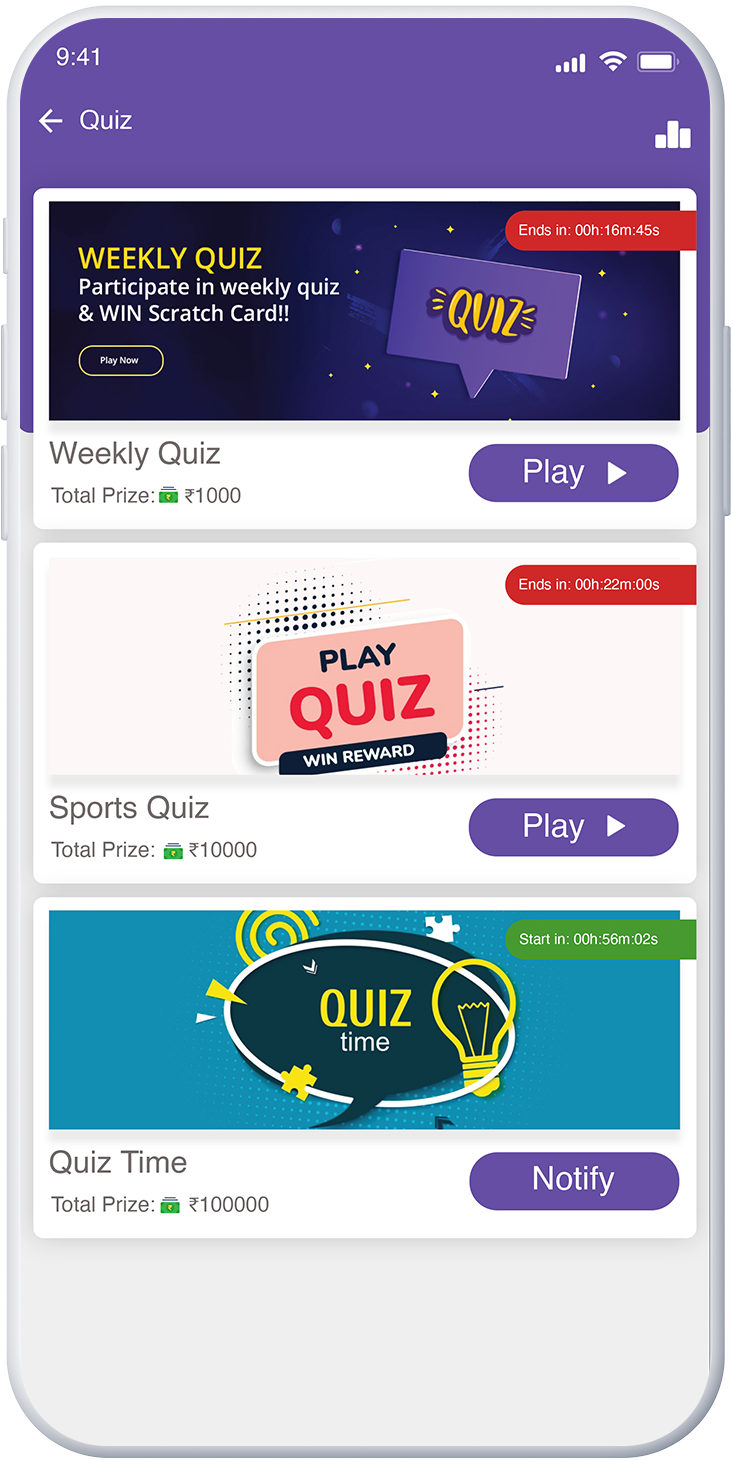
Flyy.sharedInstance.openQuizListPage()
[flyy openQuizListPage];
Stamps Screen
To open stamps screen for user.
Flyy.sharedInstance.openFlyyStampsPage(navigationController: UINavigationController)
[flyy openFlyyStampsPageWithNavigationController:(UINavigationController * _Nonnull)];
Invite Detail Screen
To open the invite detail screen for user.
Flyy.sharedInstance.openInviteDetailsPage(offerId: Int)
[flyy openInviteDetailsPageWithOfferId:(NSInteger)];
Invite And Earn Screen
To open the invite and earn screen for the user.
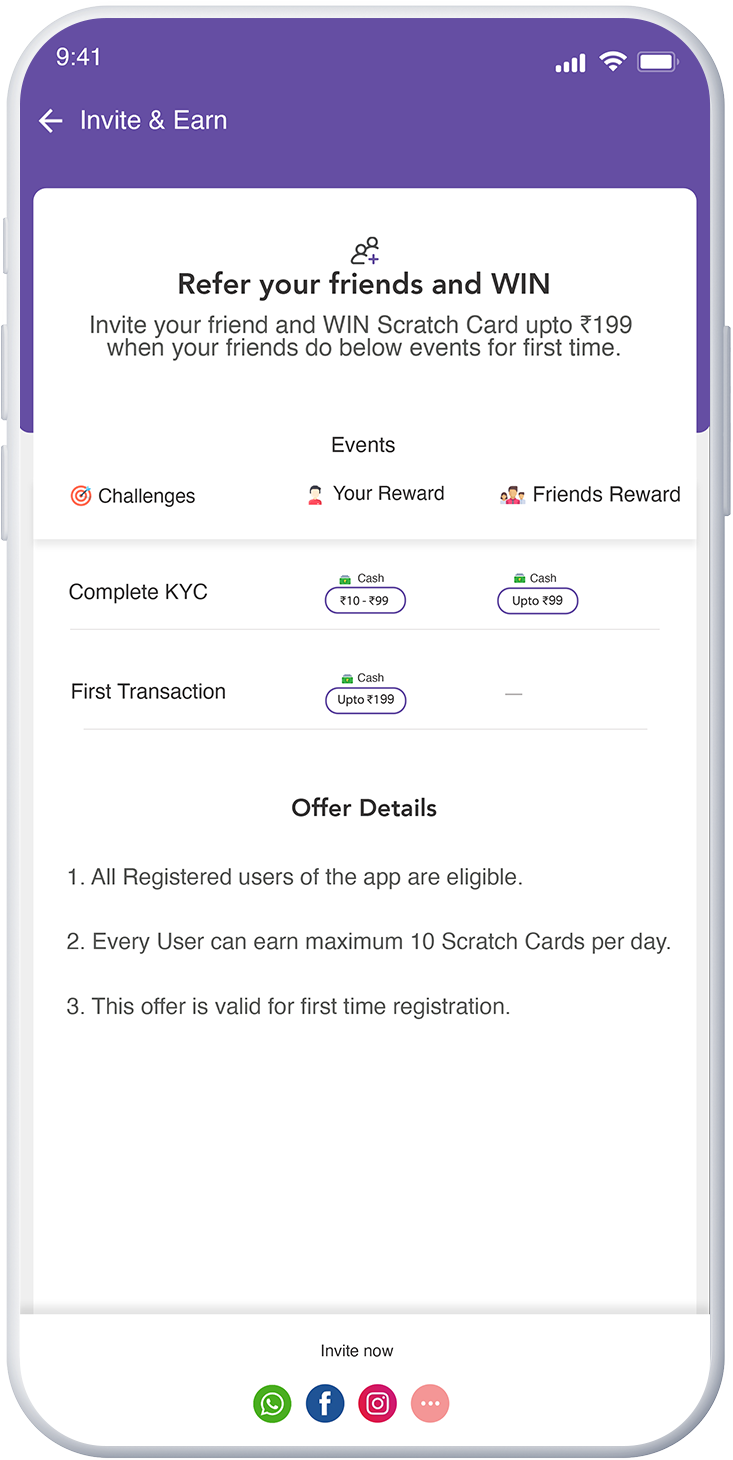
//Provide 0 to view all offers
Flyy.sharedInstance.openInviteAndEarnPage(offerId: Int)
[flyy openInviteAndEarnPageWithOfferId:(NSInteger)];
Custom Invite and Earn Screen
To open a custom invite and earn screen for the user.
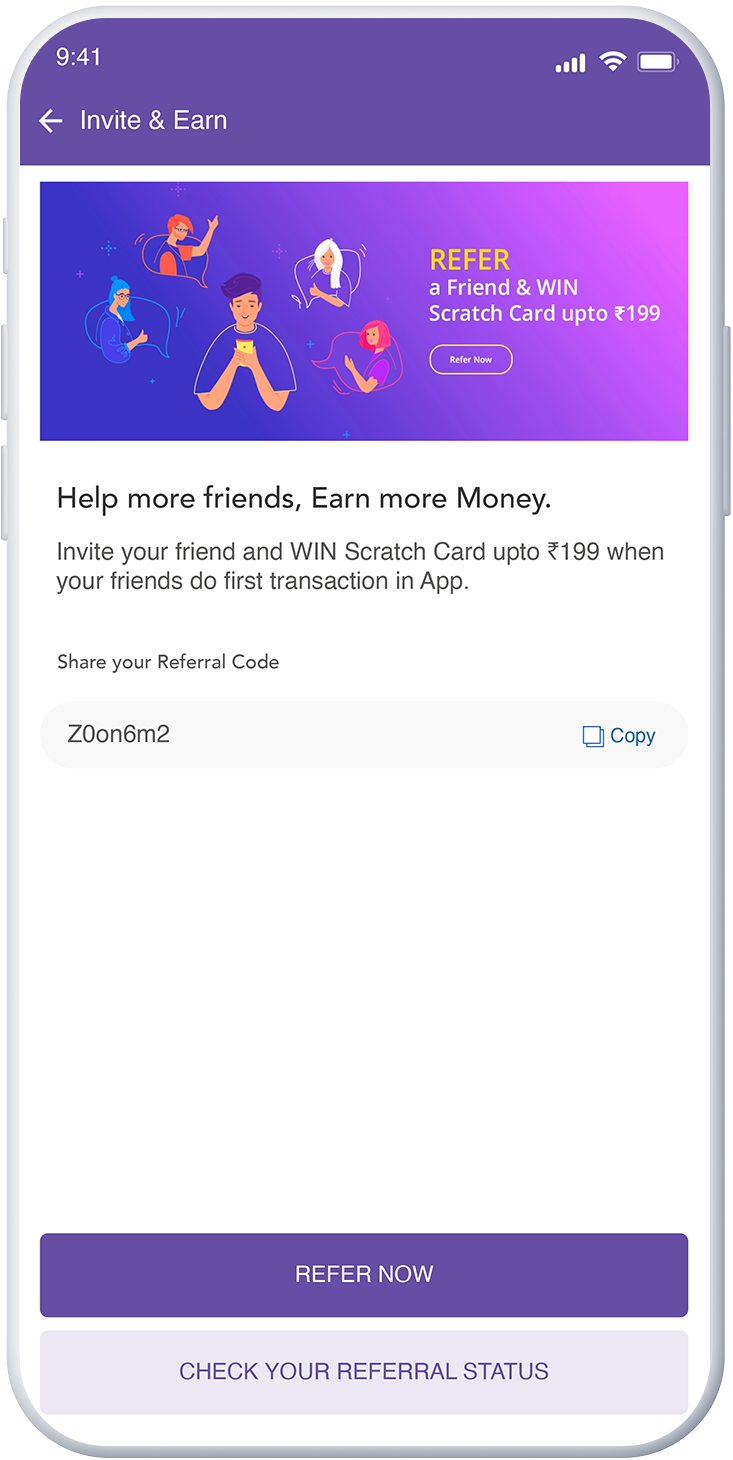
Flyy.sharedInstance.openFlyyCustomInviteAndEarnVC(offerId: Int, headerColor: "#FFFFFF")
[flyy openFlyyCustomInviteAndEarnVCWithOfferId:0 headerColor:@"#FFFFFF"];
Challenge Details Screen
To open the challenge details screen for the user.
Flyy.sharedInstance.openFlyyChallengesPage(offerId: Int)
[flyy openFlyyChallengesPageWithOfferId:(NSInteger)];
Bonanza Screen
To open a bonanza details screen for user.
Flyy.sharedInstance.openBonanzaPage()
[flyy openBonanzaPage];
Additional Methods
Set Theme Colors
The Flyy SDK inherits theme colors which are provided to the setThemeColor method. The setThemeColor method must be called immediately after the init method is called.
//Parameters to be passed are color_primary and color_primary_dark
Flyy.sharedInstance.setThemeColor(colorPrimary: "#FF5733", colorPrimaryDark: "#842C19")
//Parameters to be passed are color_primary and color_primary_dark
[flyy setThemeColorWithColorPrimary:@"#FF5733" colorPrimaryDark:@"#842C19"];
Set Name
This will be shown in the screens wherever username is displayed.
Flyy.sharedInstance.setUserName(userName: "<user_name>")
[flyy setUserNameWithUserName:@"<user_name>"];
Set User Token
To increase security for your users you can set unique user token in the following manner instead of setUser() method.
Flyy.sharedInstance.setUserToken(userToken:”<user_token>”)
[flyy setUserTokenWithUserToken:@"<user_token>"];
Set User Token along with Segment ID
To increase security for your users you can set unique user token along with segment id in the following manner instead of setUser() method
Flyy.sharedInstance.setUserToken(userToken:”<user_token>”, segmentId:“<segment_id>”)
[flyy setUserTokenWithUserToken:@"<user_token>" segmentId:@"<segment_id>"];
Set New User Token
To increase security for your users you can set unique new user token for new registered users in the following manner instead of setNewUser() method
Flyy.sharedInstance.setNewUserToken(userToken:”<user_token>”)
[flyy setNewUserTokenWithUserToken:@"<user_token>"];
Set New User Token along with Segment ID
To increase security for your users you can set unique new user token for new registered users along with segment id in the following manner instead of setNewUser() method
Flyy.sharedInstance.setNewUserToken(userToken:”<user_token>”, segmentId:“<segment_id>”)
[flyy setNewUserTokenWithUserToken:@"<user_token>" segmentId:@"<segment_id>"];
Set Bank Details
You can use the following method to set user redemption details, which takes following params,
- Account number - bank account number
- IFSC code
- Name
Flyy.sharedInstance.setBankDetails(accountNumber: “”, ifscCode: “”, accountHolderName: “”)
[flyy setBankDetailsWithAccountNumber:@"account_number" ifscCode:@"ifsc_code" accountHolderName:@"holder_name"];
Set UPI Details
You can use the following method to set user redemption details, which takes following params,
- UPI ID
Flyy.sharedInstance.setUPI(upiId: "")
[flyy setUPIWithUpiId:@"upi_id"];
Send Events
Sending User Event with API
The
sendEvent
method should only be used for testing on the Staging environment.
For production, use the Sending User Event API call from your backend instead. AllsendEvent
events generating from the front-end are ignored by the Flyy's reward system.
Values can either be sent in the form of key and value or as a json object.
Flyy.sharedInstance.sendEvent(key: "<key>", value: "<value>")
//OR
Flyy.sharedInstance.sendEvent(key: "<key>", value: "<json_any>")
[flyy sendEventWithKey:@"key" value:@"value"];
//OR
NSDictionary *json = @{@"purchase": @500};
[flyy sendEventWithJsonWithKey:@"event" value:json];
Set Referral Code
To set referral code, please call the following function
Flyy.sharedInstance.setReferralCode(referralCode: "tuwfj42")
[flyy setReferralCodeWithReferralCode:@"tuwfj42"];
Get Referral Code
To get referral code, please call the following function
let referralCode = Flyy.sharedInstance.getReferralCode()
NSString *referralCode = [flyy getReferralCode];
Verify Referral Code
To verify if referral code is valid or not, please call the following function
Flyy.sharedInstance.verifyReferralCode(referralCode: "tuwfj42", onComplete: { (success, referralCode)
-> Void in
if(success) {
print(referralCode)
}
})
[flyy verifyReferralCodeWithReferralCode:@"referral_code" onComplete:^(BOOL isValid, NSString* referralUrl) {
if (isValid) {
NSLog(@"Referral Code: ", referralCode);
}
}];
Show the Notification Popup
Use the below code to show a default Flyy notification popup.
Flyy.sharedInstance.displayNotificationPopupView(title: String, body: String)
[flyy displayNotificationPopupViewWithTitle:@"title" body:@"body_text"];
Show Reward Won Popup
Use the below code to show the reward won popup.
Flyy.sharedInstance.displayNotificationToastView(title: String, buttonText: String, deeplink: String, duration: Int)
[flyy displayNotificationToastViewWithTitle:@"title" buttonText:@"button_text" deeplink:(@"deeplink") duration:(NSInteger)];
Show Reward Won Scratch Card Popup
Use the below code to show the reward won scratch card popup.
Flyy.sharedInstance.displayNotificationScratchView(title: String, body: String, refNum: String)
[flyy displayNotificationScratchViewWithTitle:@"title" body:@"body" refNum:@"ref_num"];
Get Share Data
Use the below code to get share data
Flyy.sharedInstance.getShareData(onComplete: { (success, referralDetails) -> Void in
if(success) {
referralDetails[0] //to get referral link
referralDetails[1] //to get referral message
referralDetails[2] //to get share message
}
})
[flyy getShareDataOnComplete: ^(BOOL success, NSArray<NSString *> *referralDetails) {
NSLog(@"%@", referralDetails[0]); //referral link
NSLog(@"%@", referralDetails[1]); //referral message
NSLog(@"%@", referralDetails[2]); //share message
}];
Get Referral Count
Use the below code to get referral count.
Flyy.sharedInstance.getReferralCount(onComplete: {(success, referralCount) -> Void in
if(success) {
referralCount //referral_count
}
})
[flyy getReferralCountOnComplete:^(BOOL success, NSInteger referralCount) {
NSLog(@"%ld", (long)referralCount);
}];
Get Scratch Card Count
Use the below code to get scratch card count.
Flyy.sharedInstance.getScratchCardCount(onComplete: {
(success, scratchCardCountData) -> Void in
if(success) {
print("Total SC Count \(scratchCardCountData[0])")
print("Scratched SC Count \(scratchCardCountData[1])")
print("UnScratched SC Count \(scratchCardCountData[2])")
print("Locked SC Count \(scratchCardCountData[3])")
}
})
[flyy getScratchCardCountOnComplete: ^(BOOL success, NSArray<NSNumber *> *scratchCardCountData) {
NSLog(@"%@", scratchCardCountData[0]); //total sc count
NSLog(@"%@", scratchCardCountData[1]); //scratched sc count
NSLog(@"%@", scratchCardCountData[2]); //unscrached sc count
NSLog(@"%@", scratchCardCountData[3]); //locled sc count
}];
Get Previous Leaderboard Winner
Use the below code to get previous leaderboard winners.
Flyy.sharedInstance.getPreviousLeaderboardWinners(tag: "test", onComplete: {
(success, message, participantsCount, winnersArray, previousWinnersArray) -> Void in
if(success) {
print("Message \(message)")
print("Participants Count \(participantsCount)")
print(winnersArray)
print(previousWinnersArray)
let data = winnersArray.data(using: .utf8)!
do {
if let jsonArray = try JSONSerialization.jsonObject(with: data, options : .allowFragments)
as? [Dictionary<String,Any>]
{
print(jsonArray)
print(jsonArray[0]["rank"])
} else {
print("bad json")
}
} catch let error as NSError {
print(error)
}
} else {
print(message)
}
})
[flyy getPreviousLeaderboardWinnersWithTag:@"test" onComplete:^(BOOL success, NSString* message, NSInteger participantsCount, NSString* winners, NSString* previousWinners) {}];
Get Leaderboard Participants
Use the below code to get leaderboard participants.
Flyy.sharedInstance.getLeaderboardParticipants(tag: "test", onComplete: {
(success, message, participantsCount, winnersArray, previousWinnersArray) -> Void in
if(success) {
print("Message \(message)")
print("Participants Count \(participantsCount)")
print(winnersArray)
print(previousWinnersArray)
let data = winnersArray.data(using: .utf8)!
do {
if let jsonArray = try JSONSerialization.jsonObject(with: data, options : .allowFragments)
as? [Dictionary<String,Any>]
{
print(jsonArray)
print(jsonArray[0]["rank"])
} else {
print("bad json")
}
} catch let error as NSError {
print(error)
}
} else {
print(message)
}
})
[flyy getLeaderboardParticipantsWithTag:@"test" onComplete:^(BOOL success, NSString* message, NSInteger participantsCount, NSString* winners, NSString* previousWinners) {}];
Get Wallet Balance
Use the below code to get wallet balance.
Flyy.sharedInstance.getWalletBalance(walletLabel: "points", onComplete: {
(success, walletBalanceData) -> Void in
if(success) {
print("Balance \(walletBalanceData[0])")
print("Total Credit \(walletBalanceData[1])")
print("Total Debit \(walletBalanceData[2])")
}
})
[flyy getWalletBalanceWithWalletLabel:@"cash" onComplete:^(BOOL success, NSArray<NSNumber *> *walletBalance) {
NSLog(@"%@", walletBalance[0]); //balance
NSLog(@"%@", walletBalance[1]); //total credit
NSLog(@"%@", walletBalance[2]); //total debit
}];
Get Referrer Details
Use the below code to get referrer details.
Flyy.sharedInstance.getReferrerDetails(onComplete: {
(success, referralData) -> Void in
if(success) {
print("Name \(referralData[0])")
print("Ext UID \(referralData[1])")
print("Extra Data \(referralData[2])")
}
})
[flyy getReferrerDetailsOnComplete:^(BOOL success, NSArray<NSString *> *referrerDetailsData) {
NSLog(@"%@", referrerDetailsData[0]); //name
NSLog(@"%@", referrerDetailsData[1]); //ext uid
NSLog(@"%@", referrerDetailsData[2]); //extra data
}];
Get Offers Count
Use the below code to get offers count.
Flyy.sharedInstance.getOffersCount(onComplete: {
(success, offersCountData) -> Void in
if(success) {
print("Live offers Count \(offersCountData[0])")
print("Participated Offers Count \(offersCountData[1])")
}
})
[flyy getOffersCountOnComplete: ^(BOOL success, NSArray<NSNumber *> *offersCount) {
NSLog(@"%@", offersCount[0]); //live offers count
NSLog(@"%@", offersCount[1]); //participated offers count
}];
Advanced Implementation
Set SDK Closed Listener
To set flyy sdk closed listener
Flyy.sharedInstance.setSDKClosedListener(flyysdkclosedlistener: self)
[flyy setSDKClosedListenerWithFlyysdkclosedlistener:self];
Listen to SDK Closed Listener
extension ViewController:FlyySDKClosedListener
{
func onSDKClosed(screenName: String)
{
print(screenName)
}
}
@interface ViewController ()<FlyySDKClosedListener> {}
Change the Confetti Colors
Use the below code to change the confetti color which gets shown when you win a ScratchCard.
var confettiColors = [UIColor] ()
confettiColors.append(UIColor(red: 147/255, green: 255/255, blue:99/255, alpha: 1.0))
confettiColors.append(UIColor(red: 100/255, green: 150/255, blue:50/255, alpha: 1.0))
confettiColors.append(UIColor(red: 160/255, green: 50/255, blue:255/255, alpha: 1.0))
Flyy.sharedInstance.setConfettiColor(confettiColor: confettiColors)
Set Custom Domain
Use the below code to set the custom Domain name for Flyy SDK. Ensure you do not add https:// with the Domain.
Flyy.sharedInstance.setBaseFlyyDomain(domain: "www.xyz.com")
Flyy.sharedInstance.setBaseStageFlyyDomain(domain: "www.xyz.com")
[flyy setBaseFlyyDomainWithDomain:@"www.xyz.com"];
[flyy setBaseStageFlyyDomainWithDomain:@"www.xyz.com"];
Add User to a Segment
To add a user to a specific segment. If you are adding a user to an existing segment, you can pass null in segment_title. If the user segment is not present it will create a new one.
Flyy.sharedInstance.addUserToSegment(segmentTitle: "segment_title", segmentKey: "segment_key",
onComplete: {
(success) -> Void in
if(success) {}
})
[flyy addUserToSegmentWithSegmentTitle:segmentTitle segmentKey:segmentKey onComplete: ^(BOOL success) {}];
Updated over 2 years ago