Campaign Guides
Invite & Earn
The following documentation contains guides for implementing and testing the Invite & Earn Campaign in a Flutter Project.
Prerequisites
• App setup with the Flyy SDK. Setup Guide.
• An Invite & Earn Campaign running.
Overview
The referral process starts when the Referrer (User A) shares their referral link from the Offers Page on the app.
After User A has shared the link containing the Referral code, the Referee (User B) can apply the referrer's code in one of the following two ways.
- By manually typing in the referral code.
- Automatically when the app is downloaded and installed from App Store or Play Store using the referral link.
Next, the user Signs Up and performs the Event, say, updating their KYC, which will complete this process and distribute the rewards.
The above makes up the complete referral process.
The referral process on User B's device is summarized in the diagram below. The developer should ensure that User B executes these steps in the exact order for a successful referral.
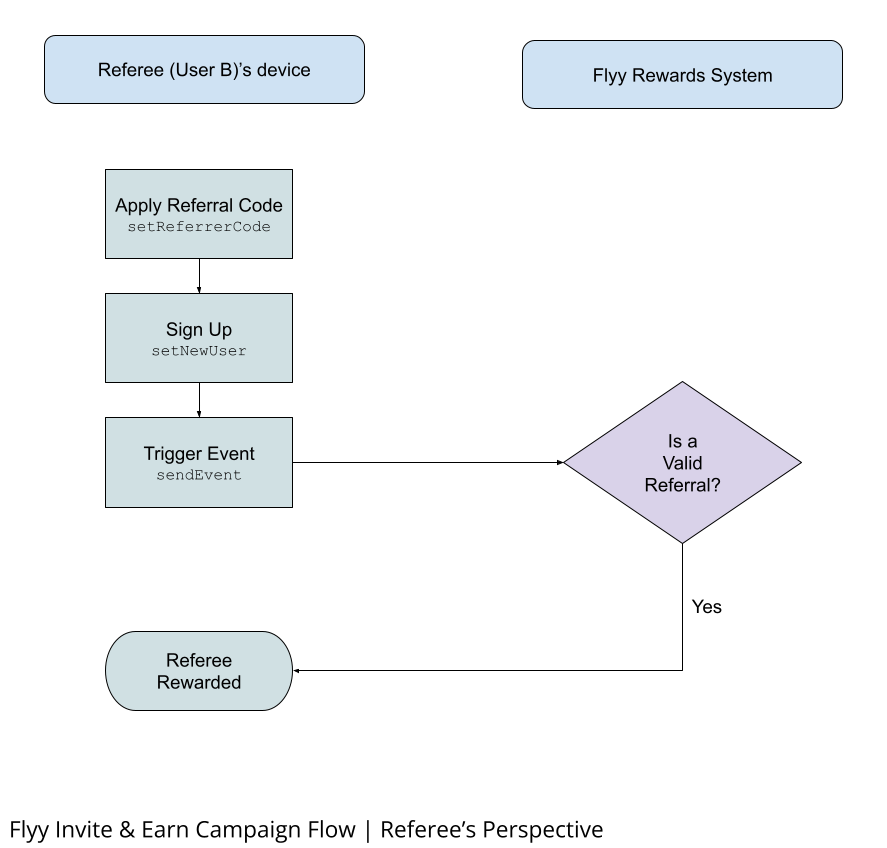
Implementation
Step 1: Initialize with initFlyySDKWithReferralCallback
This step takes care of one of the two ways a referral code is automatically applied. Use the initFlyySDKWithReferralCallback
to initialize the SDK instead of the initFlyySDK
method.
iOS 14.5+
Due to Apple's App Tracking Transparency Policy introduced from iOS 14.5, referral links might not work. Users should therefore be allowed to manually set the referral code as explained in Step 2 below.
Use the then
callback to perform additional UI-related actions, such as verifying the incoming referrer code. Do not use the await
keyword with the initFlyySDKWithReferralCallback
as it may never return a Future.
The referrer's code is automatically applied.
FlyyFlutterPlugin.initFlyySDKWithReferralCallback(
partnerId, FlyyFlutterPlugin.STAGE)
.then((value) {
// value is a List -- of which the first element is the Referral Code.
referralCode = value[0];
setState(() {});
});
Step 2: Set Referral Code
This step takes care of the other way where the referral code is manually applied.
If the user is required to apply the referral code manually, the setFlyyReferralCode
method should be used as follows.
However, note that the setFlyyReferralCode
method must be called before callingsetNewUser
.
Map<String, dynamic> response = await FlyyFlutterPlugin.verifyReferralCode(referralCode);
bool isValid = response["is_valid"];
if (isValid) {
FlyyFlutterPlugin.setFlyyReferralCode(referralCode);
}
Step 3: Sign Up
When working with an Acquisition Module campaign, it is necessary that a new user registers with the setFlyyNewUser
method instead of setFlyyUser
.
FlyyFlutterPlugin.setFlyyNewUser(userId);
Step 4: Trigger Event
The final step to completing the referral process is triggering an event. The rewards will be distributed only after the campaign event is sent.
//Note: Only use the sendEvent method in Staging. For production, make
//an API call from your backend.
//See: https://docs.theflyy.com/docs/create-and-track-your-first-offer#send-event-on-checkout
FlyyFlutterPlugin.sendEvent(eventId, "true");
Variant Campaign Event
Follow this step only to implement the variant campaign.
From the implementation standpoint, there is just 1 difference when working with Variant Campaigns: the sendEvent
method expects Event Data as a JSON object.
The below code sample is about the Variant Campaign created here from the Dashboard.
final Map<String, String> json = {"purchase": "500"};
FlyyFlutterPlugin.sendJSONEvent(eventId, json);
After the 4th step, the Flyy Rewards System sends out a scratch card to the Referrer (User A) and Referee (User B).
The Flyy Rewards System performs a few checks to determine if the new users are eligible before giving out the rewards. You may run into these safeguards while testing the Invite & Earn implementation. If you do, you may find possible resolutions in the Test Invite & Earn and the Troubleshooting sections.
Test Invite & Earn
Prerequisites
- Two devices (or two emulators with Google Play Services) — one for User A and one for User B.
Use the below 4 steps to test this offer in your own app or download the sample project from GitHub.
The Flyy Rewards System requires that the mobile device used for registering a user should not have been used before for registering any other user. See the Troubleshooting section to learn how to remove users previously registered using the same device.
Test with your app
On User A's device
- Sign Up and check the Offers Page screen to get the Referral Code.
The referral link will look something like this: https://stage.flyy.in/C8UFPBE
.
In this example link C8UFPBE is user A's referral code.
On User B's device
- Apply the Referral Code shared by User A.
- Perform Sign Up.
- Perform the action on User B's device that triggers the "kyc_done" event.
After the event is triggered, the Flyy Rewards System will check if this is a genuine referral and give out the reward containing a Scratch Card and notification to User A and B's devices.
Test with the GitHub Project
Download the Flutter project from GitHub.
Set up FCM notifications and App Store app and Play Store app redirects before starting the test.
Set up FCM Notifications
In your staging dashboard, go to Settings > SDK Keys and provide the following server key:
AAAAgM9X2Bw:APA91bGAcRPmTKjTGTESQfzUsqmCYBRnhQm4KnwuSoOpRKpn1OB5FEdqtF_CFWGMbIUyVIGecIjMwIdtx0XmYlo-dZjpLgtMLvYET9SRfr8uFzzXg441GbFyA8epdibsh3x3UKzD3glG
Redirect to Store Links
Find out how to perform these steps for the iOS app and Android app from these links.
Then, perform the following steps.
On User A's device
- Run the project.
- On the SDK Setup screen, type in the Package Name and Partner Id as it appears in your Staging dashboard.
- Sign Up with a new User ID.
- Tap the Invite & Earn button, open the Offer, and Share the Referral Link.
On User B's device
- Tap on the Referral Link. The link should open on the Safari browser for iOS, but it will show an error as we left the app store link field empty. For Android, the link will redirect to the App Page in the Play Store -- but do not download the app.
- Run the app.
- Type in the Package Name and Partner Id. Click Next. An alert message indicating whether the Referral Code is valid or invalid will be displayed. If the Referral Code is valid, it is applied without needing any further steps.
- If the referral code is invalid, manually enter the referral code on the Sign-Up screen.
- Sign Up with a new User ID.
- On the Home screen, tap the Send Event button. This button is calling the
sendEvent
method with "kyc_done" as the event key in its parameter.
After tapping on the Send Event button, the Flyy Rewards System determines whether to give out the reward or not and sends a Scratch Card and notification to User A and B's devices.
Troubleshooting
If you are not receiving rewards during testing. See the common problems below.
Multiple Devices with the same Device ID
Users A and B must sign up from unique devices and the same device should not exist on Flyy's dashboard before they sign up.
To verify the uniqueness of the device id, go to Settings > Playground and follow these steps:
- Perform a Search with User A's user id.
- Copy the Device Id from the search result.
- Perform another search with the copied Device Id.
- If more than 1 search results show up, delete all records with identical Device Id.
Repeat the same steps for User B.
After you have ensured that all records with the above Device Ids have been deleted, rerun the test.
Notification Issue
Go to Reports > Referrals and check if the new referral record with User A and B record is created. If the new record exists, but the devices do not receive a notification, test your app's FCM configuration.
Also, ensure if the correct Server Key has been provided at Settings > SDK Keys.
To test notifications from the dashboard.
- Go to Settings > Playground from the dashboard.
- Search by User Id.
- Click "Test FCM". Note that at least 1 Campaign must be active.
Updated almost 3 years ago